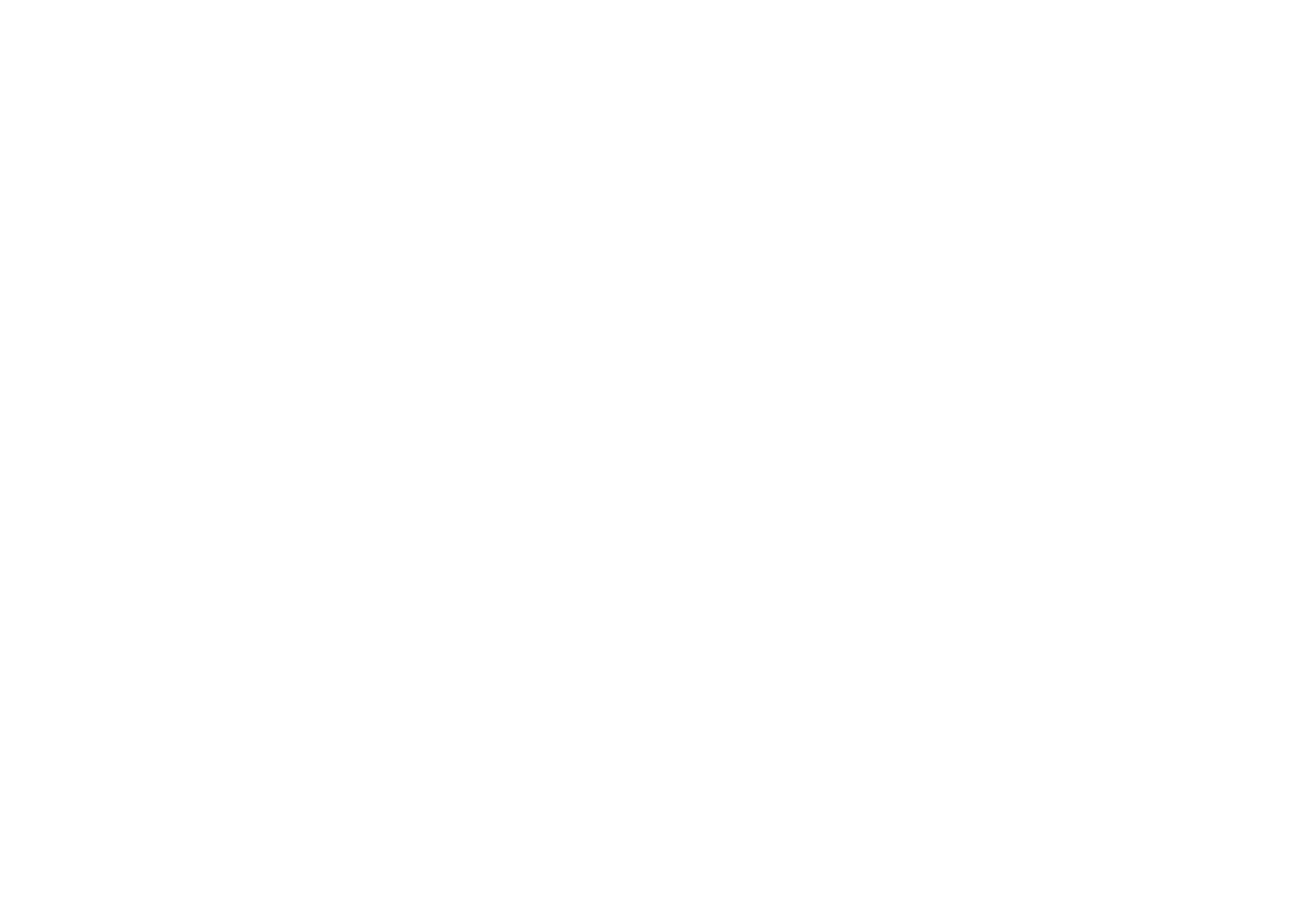
Creating Custom Post Types in WordPress Using PHP
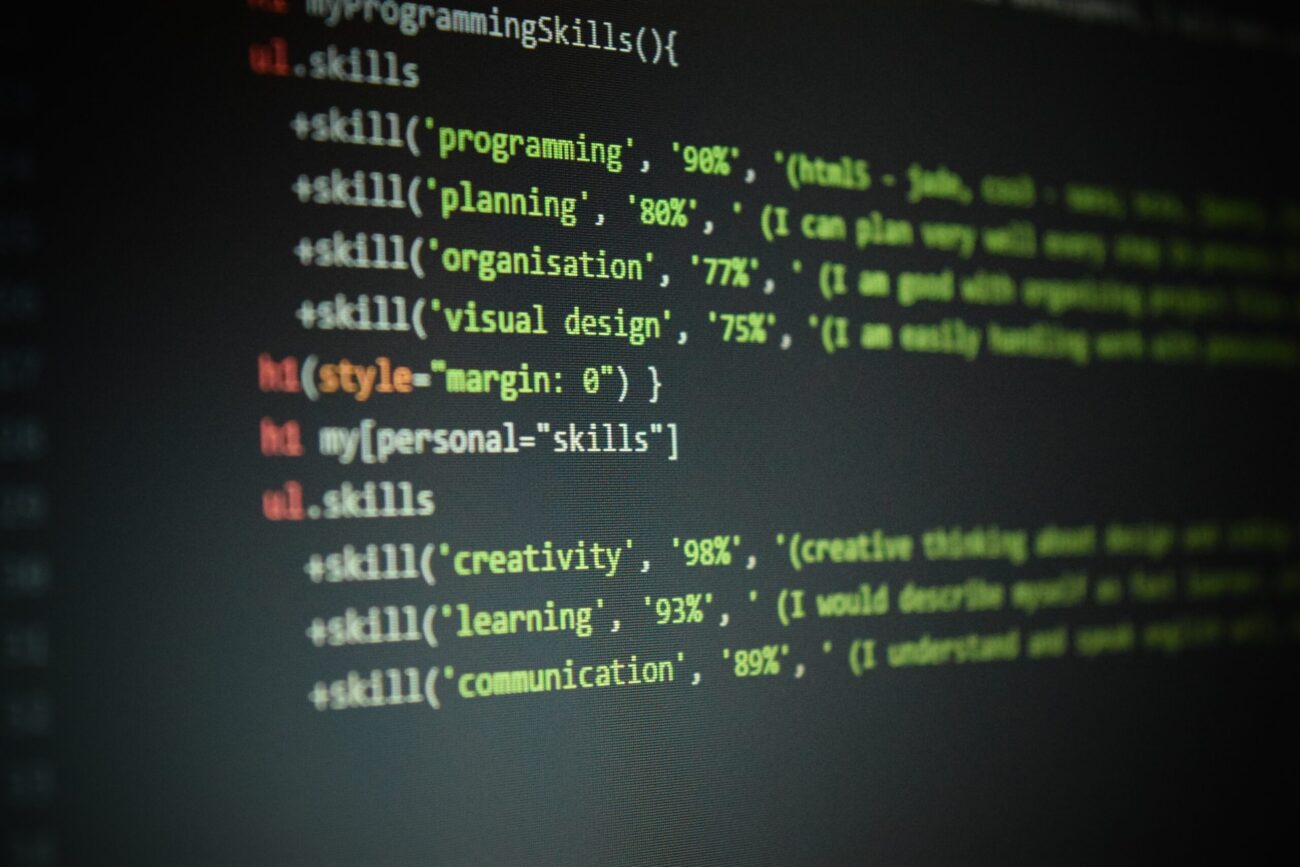
WordPress is one of the most popular platforms for building websites, and its flexibility allows developers to create various types of content. In this article, we will walk you through creating custom post types on your WordPress site using the PHP programming language. Custom post types allow you to organize content in a way that best suits your needs.
Introduction to Custom Post Types
The default post types in WordPress, such as «Posts» and «Pages,» work well for most websites. However, sometimes you may need a more specific type of content. For example, custom post types are perfect for portfolios, products, events, and more.
Advantages of using custom post types:
- Content Organization: Custom post types allow you to logically organize diverse content on your website.
- Ease of Management: Each custom post type has its own administrative panel, making it easy to add, edit, and delete content.
- Flexibility: You can define custom fields and taxonomies for your custom post types, tailoring them to your project’s specific requirements.
Creating Custom Post Types
Let’s start by creating a simple custom post type for a portfolio. Suppose you have a web agency and want to showcase your projects. You’ll need a post type called «Projects.»
Step 1: Adding Code to functions.php
Open your theme’s functions.php
file and add the following code:
// Register a custom post type for managing projects
function create_custom_post_type() {
$labels = array(
'name' => _x( 'Projects', 'post type general name', 'your-text-domain' ), // General name for the post type (plural)
'singular_name' => _x( 'Project', 'post type singular name', 'your-text-domain' ), // Singular name for the post type
'menu_name' => _x( 'Projects', 'admin menu', 'your-text-domain' ), // Menu name in the admin panel
'name_admin_bar' => _x( 'Project', 'add new on admin bar', 'your-text-domain' ), // Name displayed on admin bar
'add_new' => _x( 'Add New', 'project', 'your-text-domain' ), // Text for "Add New" button
'add_new_item' => __( 'Add New Project', 'your-text-domain' ), // Text for adding a new project
'new_item' => __( 'New Project', 'your-text-domain' ), // Text for a new project
'edit_item' => __( 'Edit Project', 'your-text-domain' ), // Text for editing a project
'view_item' => __( 'View Project', 'your-text-domain' ), // Text for viewing a project
'all_items' => __( 'All Projects', 'your-text-domain' ), // Text for "All Items" menu
'search_items' => __( 'Search Projects', 'your-text-domain' ), // Text for searching projects
'parent_item_colon' => __( 'Parent Projects:', 'your-text-domain' ), // Text for parent project
'not_found' => __( 'No projects found.', 'your-text-domain' ), // Text if no projects are found
'not_found_in_trash' => __( 'No projects found in Trash.', 'your-text-domain' ) // Text if no projects are found in the trash
);
$args = array(
'labels' => $labels, // Array of labels for the post type
'public' => true, // Whether the post type is publicly queryable and accessible
'publicly_queryable' => true, // Enable queries for this post type
'show_ui' => true, // Show UI in admin panel
'show_in_menu' => true, // Display in admin menu
'query_var' => true, // Enable query variables for this post type
'rewrite' => array( 'slug' => 'projects' ), // Permalink settings
'capability_type' => 'post', // Type of user permissions to handle posts
'has_archive' => true, // Enable archive page
'hierarchical' => false, // Posts will behave like flat items, not hierarchical
'menu_position' => null, // Position in admin menu
'menu_icon' => 'dashicons-portfolio', // Icon for the admin menu (see https://developer.wordpress.org/resource/dashicons/)
'supports' => array( 'title', 'editor', 'thumbnail', 'excerpt', 'custom-fields' ), // Supported features
'taxonomies' => array( 'category', 'post_tag' ), // Taxonomies associated with the post type
'show_in_rest' => true, // Enable REST API and Gutenberg editor support
);
// Register the new post type
register_post_type( 'projects', $args );
}
add_action( 'init', 'create_custom_post_type' );
This code uses the register_post_type
function, which allows you to define a new custom post type. In this case, we’re creating a post type called «Projects» with specific parameters.
Step 2: Updating Permalinks
To activate the new post type, you’ll need to update the permalinks. Simply go to «Settings» -> «Permalinks» and click «Save Changes.»
Step 3: Viewing the Results
After completing the above steps, you can navigate to the WordPress admin panel and see the new «Projects» section. Now you can create, edit, and delete your projects just like you would with regular posts and pages.
Step 4: Retrieving Data from Custom Post Types
To display data from the new custom post type on your website, you can use the following code:
$args = array(
'post_type' => 'projects',
'posts_per_page' => -1, // Show all projects
);
$projects_query = new WP_Query($args);
if ($projects_query->have_posts()) {
while ($projects_query->have_posts()) {
$projects_query->the_post();
// Display project data
echo '<h2>' . get_the_title() . '</h2>';
echo '<div>' . get_the_content() . '</div>';
// Additional fields, if any
}
wp_reset_postdata();
} else {
echo 'No projects found.';
}
In this example, we use WP_Query
to retrieve all posts of the «Projects» type. We then loop through each post and display the title and content of the project. You can also add the output of additional fields if you have defined them.
Conclusion
Creating custom post types is a powerful tool that allows you to tailor your website’s structure to your needs. In this article, we covered the basic steps of creating a custom post type «Projects» for a portfolio, as well as how to retrieve and display data from this post type on your website.
Use this knowledge to create unique and flexible content on your WordPress site!