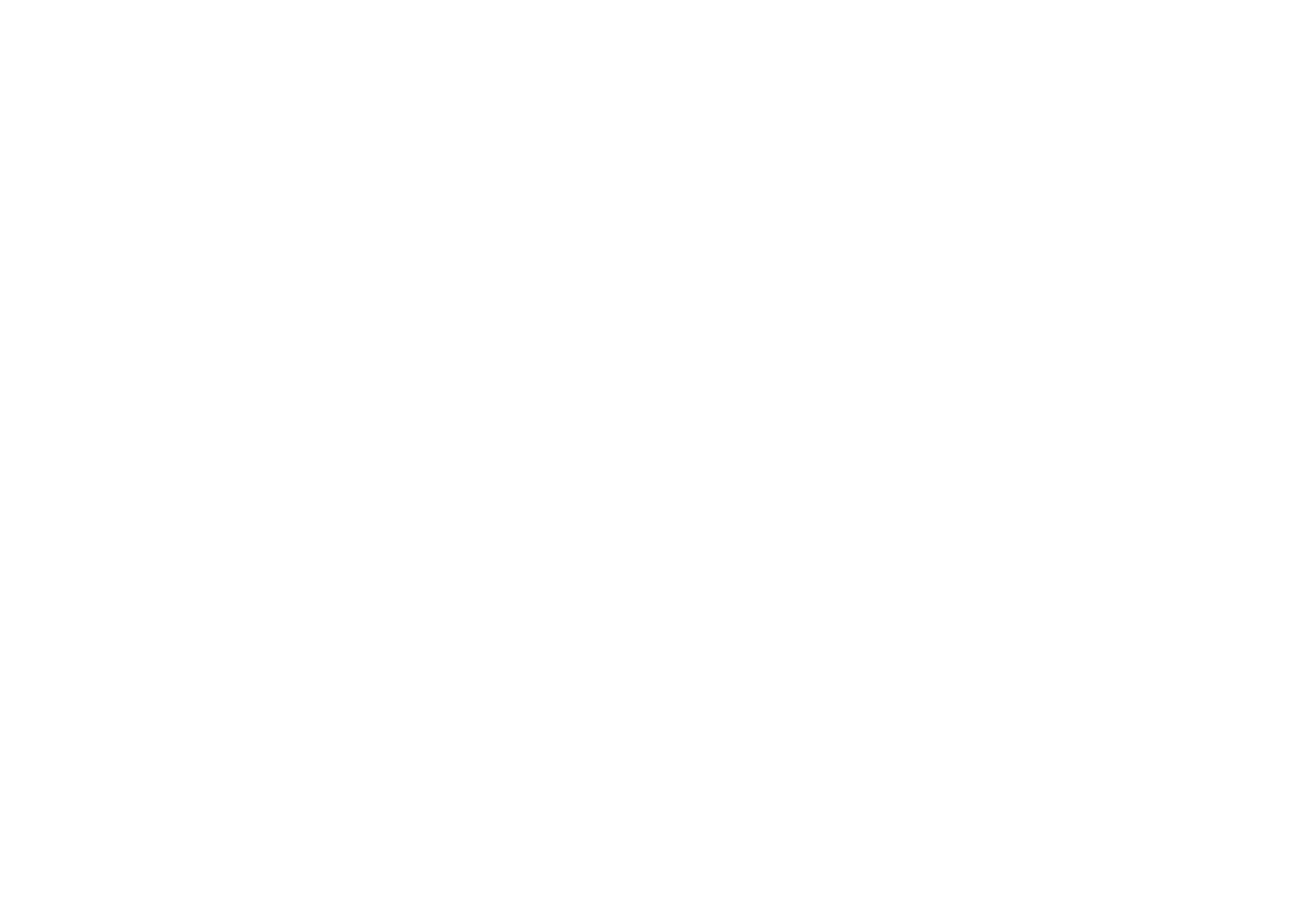
Introduction to Website Development with WordPress and PHP
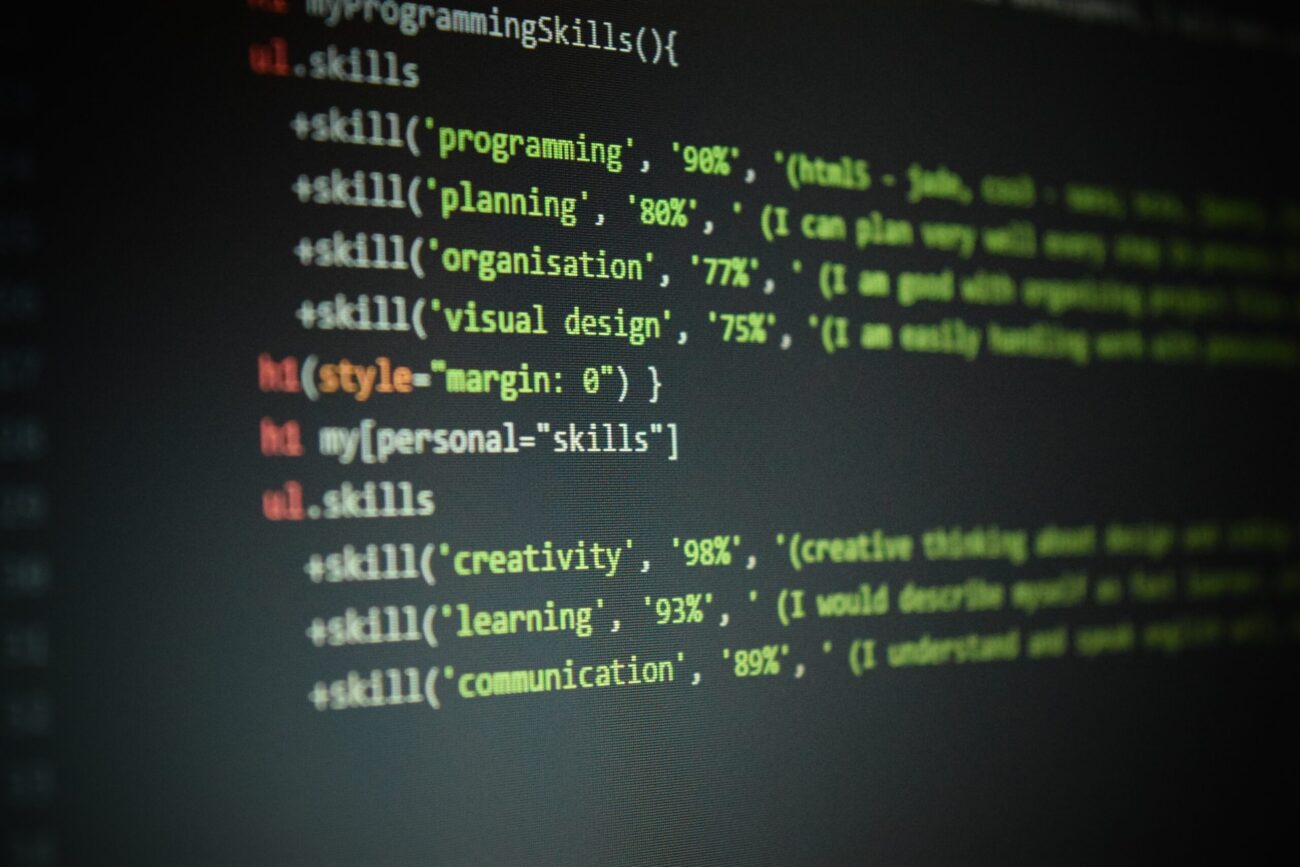
Creating websites using the WordPress platform and the PHP programming language is an engaging and powerful endeavor that allows you to build functional and creative web applications. In this article, we will explore the basics of website development using WordPress and PHP, along with providing code examples for better understanding.
Part 1: WordPress Basics
WordPress is one of the most popular platforms for creating websites and blogs. Its flexibility and user-friendliness make it an excellent choice for novice developers. Here are a few key concepts:
1.1 Installing WordPress:
The first step is to install WordPress on your hosting. Follow your hosting provider’s instructions or consult the WordPress documentation for installation guidance.
1.2 Themes and Plugins:
Themes define the appearance of your website, while plugins add additional functionality. You can choose from ready-made themes and plugins or create your own.
1.3 Creating Pages and Posts:
WordPress allows you to create static pages and blog posts. You can use the visual editor or HTML/PHP to create content.
Part 2: PHP Basics for WordPress
PHP is a server-side programming language used to create dynamic web pages. You’ll need some basic PHP knowledge to work with WordPress.
2.1 Built-in WordPress Functions:
WordPress provides a plethora of built-in functions to help you manage content and site functionality. For instance, get_header()
is used to display the site header, and the_title()
is used to display the title of a post.
2.2 Working with the Database:
WordPress stores data, such as posts and users, in a database. You can use wpdb
functions to execute database queries.
Example:
global $wpdb;
$results = $wpdb->get_results("SELECT * FROM {$wpdb->prefix}posts WHERE post_type = 'post'");
foreach ($results as $post) {
echo $post->post_title;
}
2.3 Creating Custom Shortcodes:
Shortcodes allow you to insert predefined content blocks into posts and pages. Here’s how you can create your own shortcode:
function custom_shortcode() {
return '<div class="custom-box">Hello, world!</div>';
}
add_shortcode('custom', 'custom_shortcode');
Part 3: Example WordPress Website Development using PHP
Let’s consider an example of creating a simple WordPress theme using PHP:
3.1 Theme Creation:
Create a new directory in wp-content/themes/
and name it my-custom-theme
. Inside, create a style.css
file with theme information.
Example style.css
:
/*
Theme Name: My Custom Theme
Description: Example custom theme for WordPress
Version: 1.0
Author: Your Name
*/
3.2 Creating the Main Theme File:
Create an index.php
file in your theme directory and add the following code:
<?php get_header(); ?>
<div id="content">
<?php if (have_posts()) : while (have_posts()) : the_post(); ?>
<div class="post">
<h2><?php the_title(); ?></h2>
<div class="post-content">
<?php the_content(); ?>
</div>
</div>
<?php endwhile; endif; ?>
</div>
<?php get_footer(); ?>
3.3 Styling and Images:
Create a css
directory within your theme and add a styles.css
file. You can also add images to the images
directory.
3.4 Activating the Theme:
In the WordPress admin panel, navigate to «Appearance» -> «Themes» and activate your new theme.
Conclusion:
This is just the beginning of an introduction to website development with WordPress and PHP. However, you’ve already mastered the basics of creating a WordPress theme and using PHP to manage content and functionality. Moving forward, you can explore more advanced concepts such as creating custom plugins, working with AJAX, and much more. Best of luck on your journey into the world of web development!