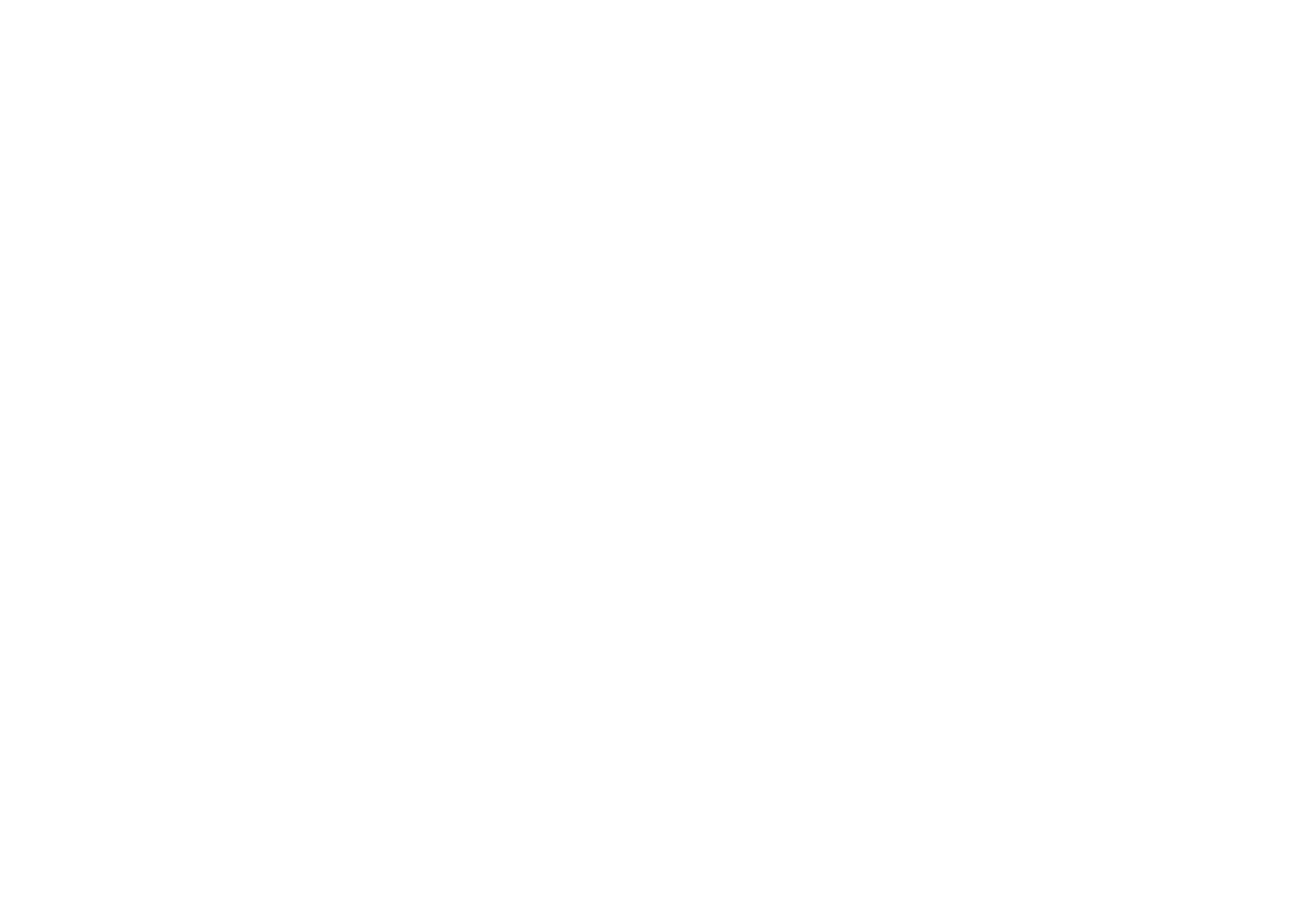
Optimizing WordPress Performance Using PHP
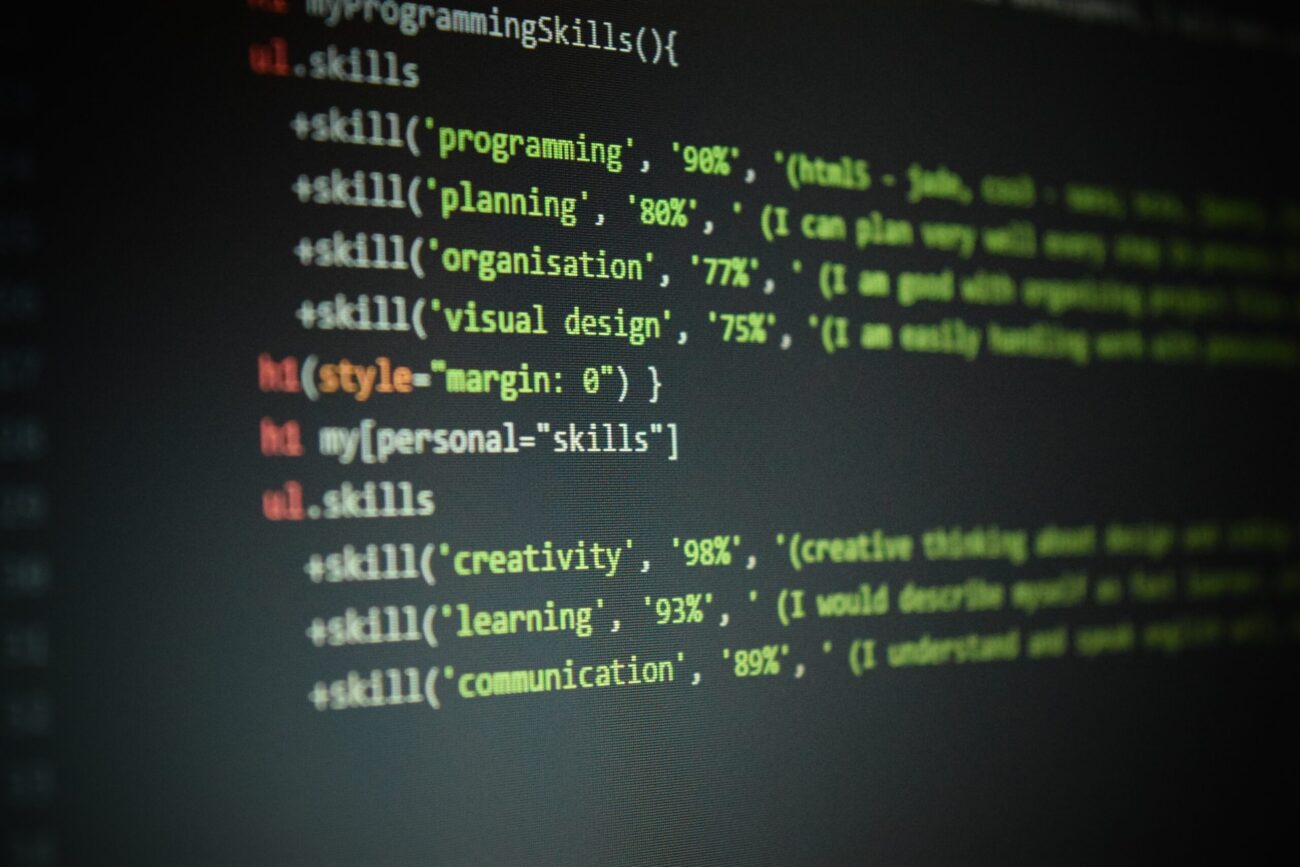
WordPress is one of the most popular platforms for creating websites and blogs, but sometimes its performance can leave something to be desired. With a constantly growing volume of content and features, performance optimization becomes a key task for webmasters and developers. In this article, we will explore how using PHP can help optimize your WordPress site’s performance. We will also provide code examples for a better understanding.
1. Caching with PHP
One of the ways to significantly improve WordPress performance is through caching. Caching allows you to store the results of queries and operations for later use, reducing server load and speeding up page loading.
Code Example:
// Enable page-level caching
if (!defined('WP_CACHE')) {
define('WP_CACHE', true);
}
// Use a cache object to store data
$cache_key = 'recent_posts';
$recent_posts = wp_cache_get($cache_key);
if (false === $recent_posts) {
$recent_posts = get_recent_posts_from_database();
wp_cache_set($cache_key, $recent_posts, 'posts', 300); // Cache for 5 minutes
}
// Use $recent_posts to display data on the page
2. Database Optimization
Efficient use of the database is another important aspect of performance optimization. PHP provides many tools for working with the database and improving its performance.
Code Example:
// Use prepared statements to prevent SQL injections
$user_id = $_GET['user_id'];
$prepared_query = $wpdb->prepare("SELECT * FROM {$wpdb->prefix}users WHERE ID = %d", $user_id);
$results = $wpdb->get_results($prepared_query);
// Use indexes to speed up queries
$wpdb->query("ALTER TABLE {$wpdb->prefix}posts ADD INDEX post_title_index (post_title)");
// Limit the number of retrieved records
$limit = 10;
$posts = $wpdb->get_results("SELECT * FROM {$wpdb->prefix}posts LIMIT $limit");
3. Query Optimization
Unnecessary and inefficient queries can slow down page loading. By using PHP, you can optimize queries and minimize server load.
Code Example:
// Select only necessary fields from the database
$posts = $wpdb->get_results("SELECT ID, post_title FROM {$wpdb->prefix}posts");
// Use caching for requests to external APIs
$weather_data = wp_cache_get('weather_data');
if (false === $weather_data) {
$weather_data = fetch_weather_data_from_api();
wp_cache_set('weather_data', $weather_data, 'api', 3600); // Cache for 1 hour
}
4. Image Optimization
Images can significantly increase page load times. PHP allows you to automate image optimization.
Code Example:
// Use the GD library to resize images
$image_path = 'path/to/image.jpg';
$thumbnail = image_resize($image_path, 300, 200, true);
// Convert images to WebP format for faster loading
if (function_exists('imagewebp')) {
$image_path = 'path/to/image.png';
$webp_image_path = 'path/to/image.webp';
$source_image = imagecreatefrompng($image_path);
imagewebp($source_image, $webp_image_path, 80);
}
Conclusion
Optimizing WordPress performance using PHP is an essential aspect of creating fast and responsive websites. In this article, we have covered some methods of caching, database optimization, query optimization, and image optimization with code examples for each. Use these tips to make your WordPress site more efficient and faster for users.