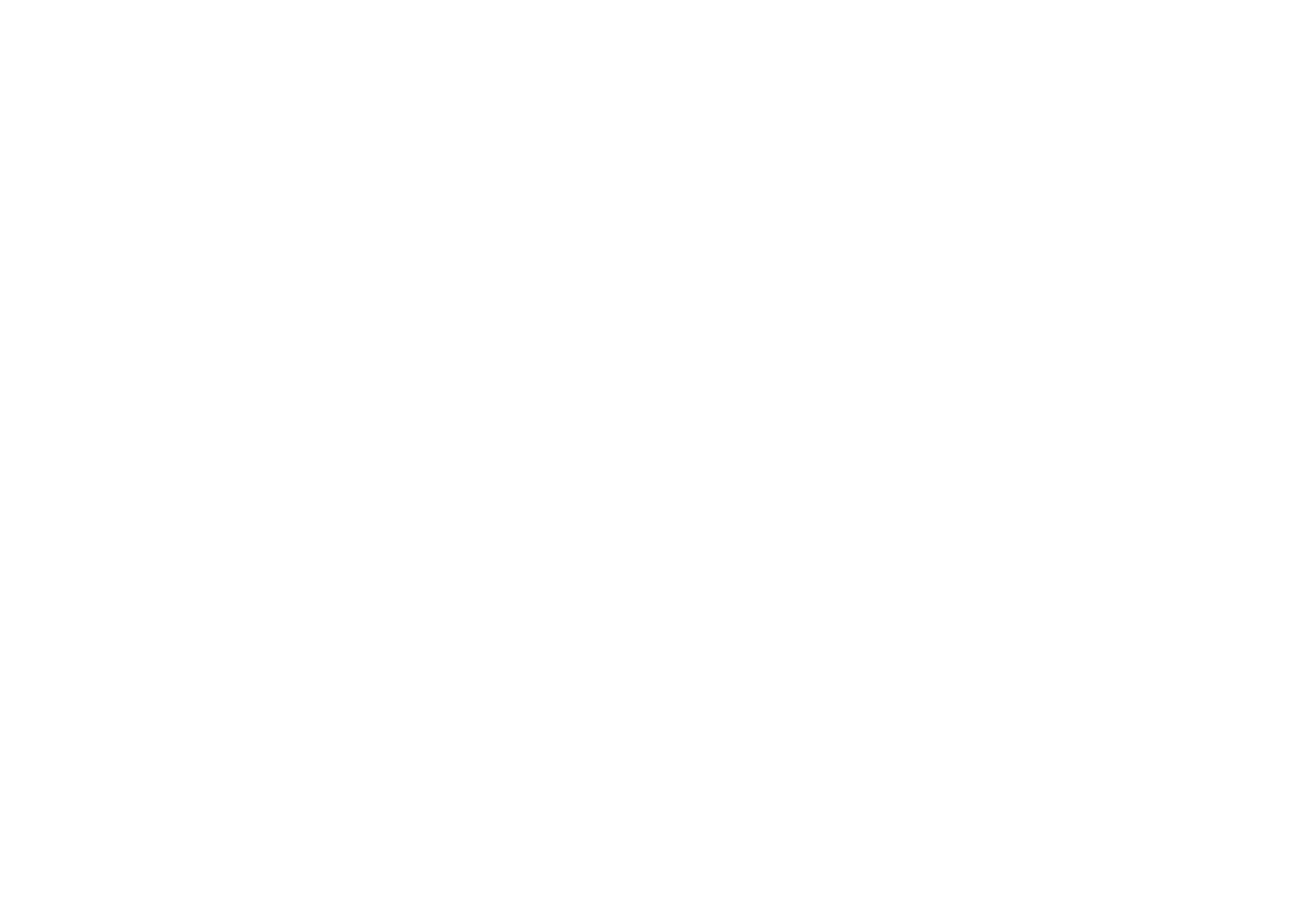
Creating Custom WordPress Themes Using PHP: A Comprehensive Guide
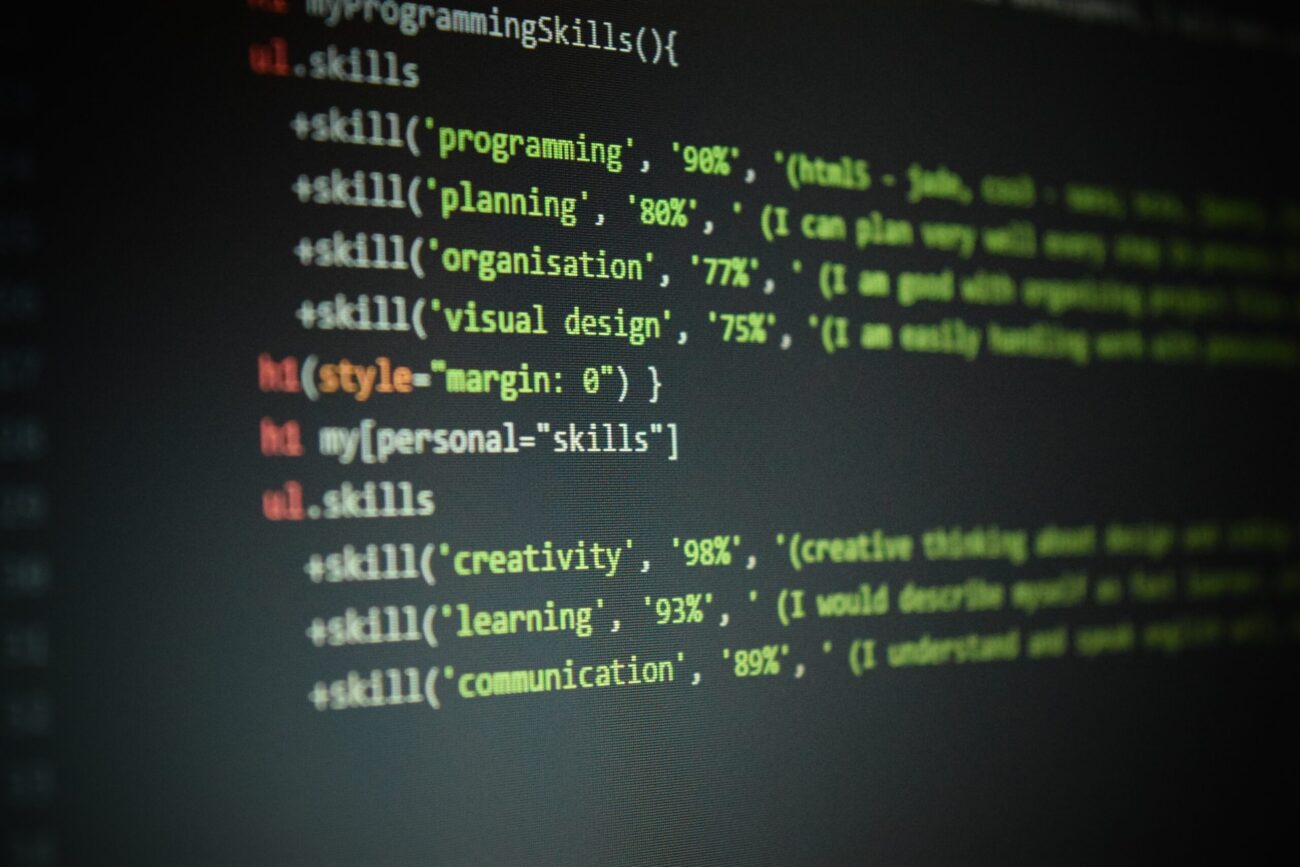
WordPress content management system is a powerful tool for creating and managing websites. One of its key features is the ability to create custom themes, which allow you to have full control over the appearance and functionality of your website. In this article, we will provide a detailed guide on creating custom WordPress themes using the PHP programming language. We’ll cover all the steps, from initial setup to adding custom templates and functions.
Step 1: Preparing for Theme Development
Before diving into theme development, ensure that you have WordPress installed on your hosting. Create a folder for your theme within the «wp-content/themes» directory and give it a unique name.
Step 2: Creating Core Theme Files
Your theme should have at least two core files: «style.css» and «index.php». In «style.css,» you’ll define information about your theme, and «index.php» will serve as the template for the main page.
Example «style.css»:
/*
Theme Name: My Custom Theme
Author: Your Name
Description: Description of your theme
Version: 1.0
*/
/* Additional styles here */
Example «index.php»:
<?php get_header(); ?>
<main id="main-content">
<?php if (have_posts()) : while (have_posts()) : the_post(); ?>
<article <?php post_class(); ?>>
<h2><a href="<?php the_permalink(); ?>"><?php the_title(); ?></a></h2>
<div class="post-content"><?php the_content(); ?></div>
</article>
<?php endwhile; endif; ?>
</main>
<?php get_sidebar(); ?>
<?php get_footer(); ?>
Step 3: Adding Custom Templates
To make your theme more flexible, you can add custom templates for different types of content, such as archive pages, blog pages, etc.
Example «archive.php»:
<?php get_header(); ?>
<main id="main-content">
<h1>Archive</h1>
<?php if (have_posts()) : while (have_posts()) : the_post(); ?>
<article <?php post_class(); ?>>
<h2><a href="<?php the_permalink(); ?>"><?php the_title(); ?></a></h2>
<div class="post-content"><?php the_excerpt(); ?></div>
</article>
<?php endwhile; endif; ?>
</main>
<?php get_sidebar(); ?>
<?php get_footer(); ?>
Step 4: Adding Custom Functions
You can expand your theme’s functionality by adding custom functions to the «functions.php» file.
Example of adding a custom function:
function custom_theme_setup() {
add_theme_support('title-tag');
add_theme_support('post-thumbnails');
register_nav_menus(array(
'primary-menu' => 'Primary Menu',
));
}
add_action('after_setup_theme', 'custom_theme_setup');
Step 5: Theme Styling
Create a «css» folder within your theme and add a «style.css» file to define your theme’s styles. You can also use CSS preprocessors like Sass or LESS.
Conclusion:
Creating custom WordPress themes using PHP is an exciting process that empowers you to have full control over your website’s appearance and functionality. In this article, we’ve covered key steps, from setup and core file creation to adding custom templates and functions. Now you have an excellent guide to create unique custom WordPress themes using PHP. Don’t limit yourself—experiment, improve, and share your creative solutions with the world!