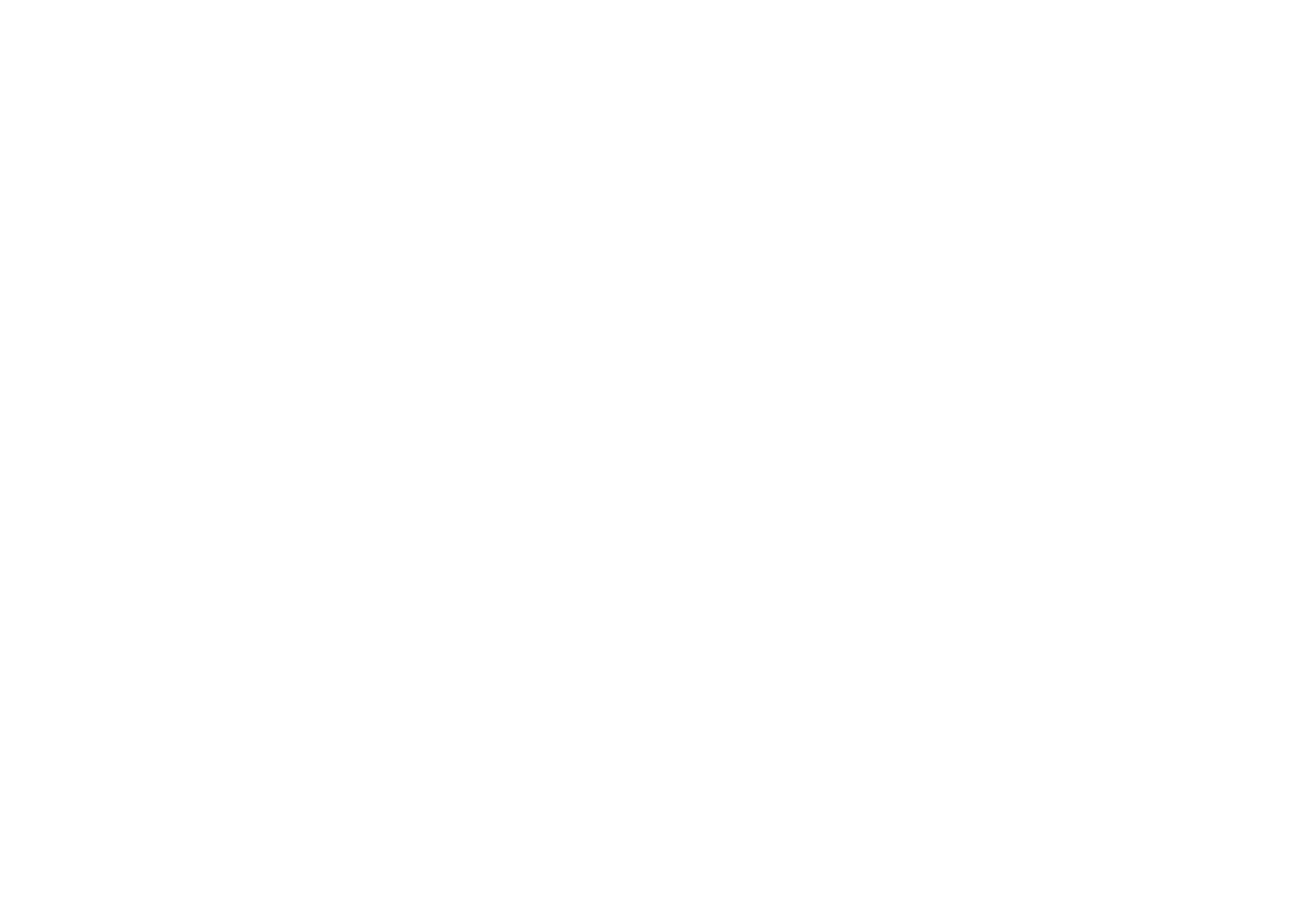
Security in WordPress and PHP Website Development
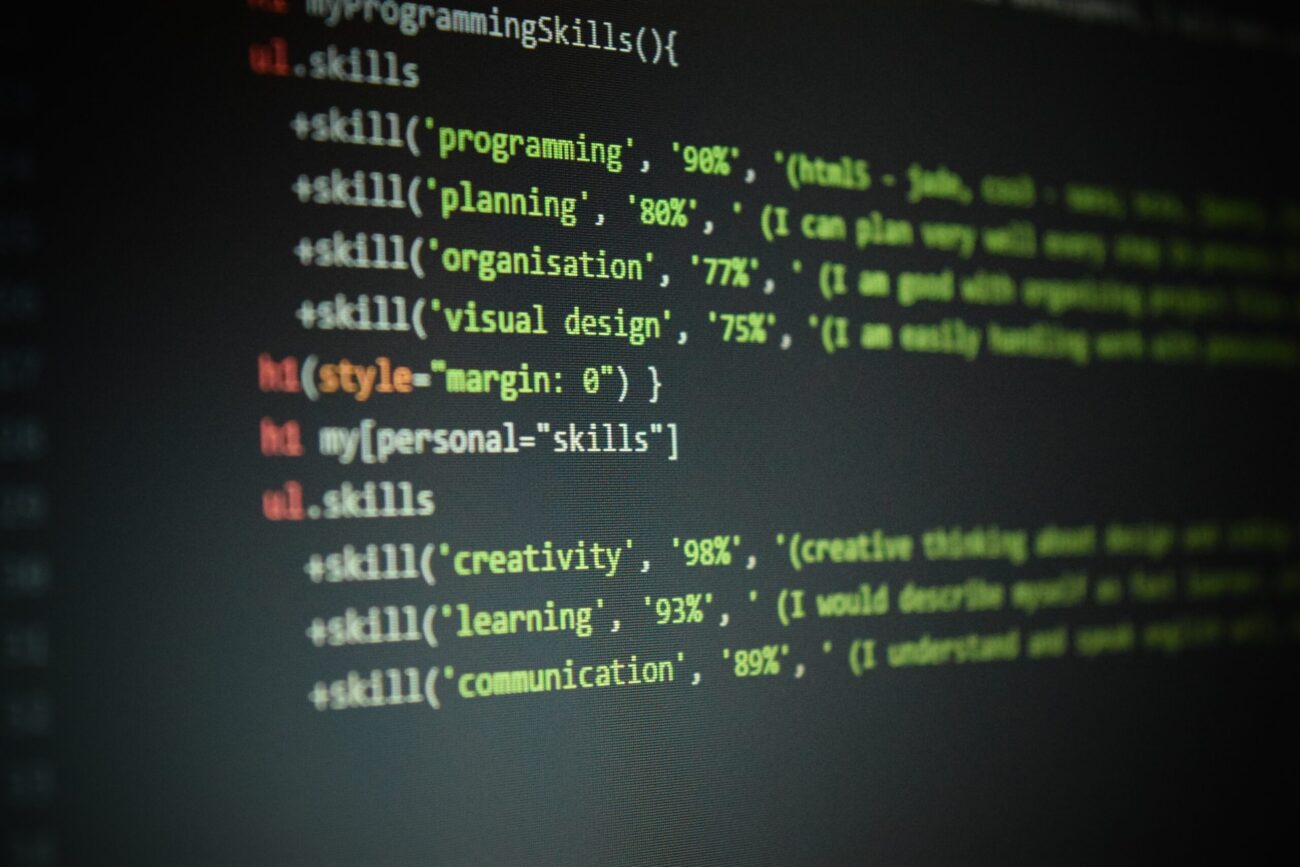
Security in web development is one of the key aspects that requires special attention when creating websites. In this article, we will explore important principles and methods for ensuring security in the development of websites on the WordPress platform using the PHP programming language. We will also provide code examples that will help you create reliable and secure web applications.
1. Software Updates
The WordPress platform and the PHP programming language are regularly updated by developers to address vulnerabilities and enhance security. Periodically check for available updates for your website and make sure you are using the latest versions of WordPress and PHP.
// Example of checking for WordPress updates
if (function_exists('wp_version_check')) {
$updates = wp_version_check();
if (!empty($updates) && $updates['response'] == 'upgrade') {
// Display a warning about available updates
}
}
2. Protection Against SQL Injections
SQL injections are a common method of attacking web applications, including WordPress websites. Use prepared statements and data escaping to prevent this vulnerability.
// Example of a prepared statement
global $wpdb;
$username = $_POST['username'];
$password = $_POST['password'];
$query = $wpdb->prepare("SELECT * FROM wp_users WHERE user_login = %s AND user_pass = %s", $username, $password);
$results = $wpdb->get_results($query);
3. Proper Handling of User Input
Always assume that user input can be dangerous and validate and filter data before using it.
// Example of email validation
$email = $_POST['email'];
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
// Actions for a valid email address
} else {
// Error handling
}
4. Protection Against Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery (CSRF) is another common type of attack. Use protection mechanisms such as generating and checking CSRF tokens.
// Example of generating and checking a CSRF token
session_start();
$csrf_token = bin2hex(random_bytes(32));
$_SESSION['csrf_token'] = $csrf_token;
// In the form
<input type="hidden" name="csrf_token" value="<?php echo $csrf_token; ?>">
// When processing the request
if ($_POST['csrf_token'] === $_SESSION['csrf_token']) {
// Actions for a valid CSRF token
} else {
// CSRF error handling
}
5. Limiting Access Rights
Do not grant unnecessary access rights to users and administrators. Use the principle of least privilege to restrict the capabilities of potential attackers.
// Example of limiting access rights
if (current_user_can('edit_posts')) {
// Actions for users with post editing rights
} else {
// Access limitation
}
6. Protection Against Malicious File Uploads
Ensure that files uploaded by users are checked for malicious code. Use whitelists of allowed file types and validate the contents of uploaded files.
// Example of checking the uploaded file type
$allowed_file_types = array('jpg', 'png', 'gif');
$uploaded_file = $_FILES['file'];
$file_extension = pathinfo($uploaded_file['name'], PATHINFO_EXTENSION);
if (in_array($file_extension, $allowed_file_types)) {
// Process the uploaded file
} else {
// File upload error
}
7. Protection of Confidential Data
Avoid storing confidential data, such as passwords, in plain text. Use hashing and salting to ensure the security of stored information.
// Example of password hashing
$password = $_POST['password'];
$salt = 'somerandomstring'; // Unique salt for each user
$hashed_password = hash('sha256', $password . $salt);
Conclusion
Ensuring security in WordPress and PHP website development is a complex but necessary task. Adhering to the principles mentioned above and using code examples will allow you to create reliable and secure web applications. Always stay updated on the latest security trends and continuously improve your knowledge to stay ahead of potential threats.