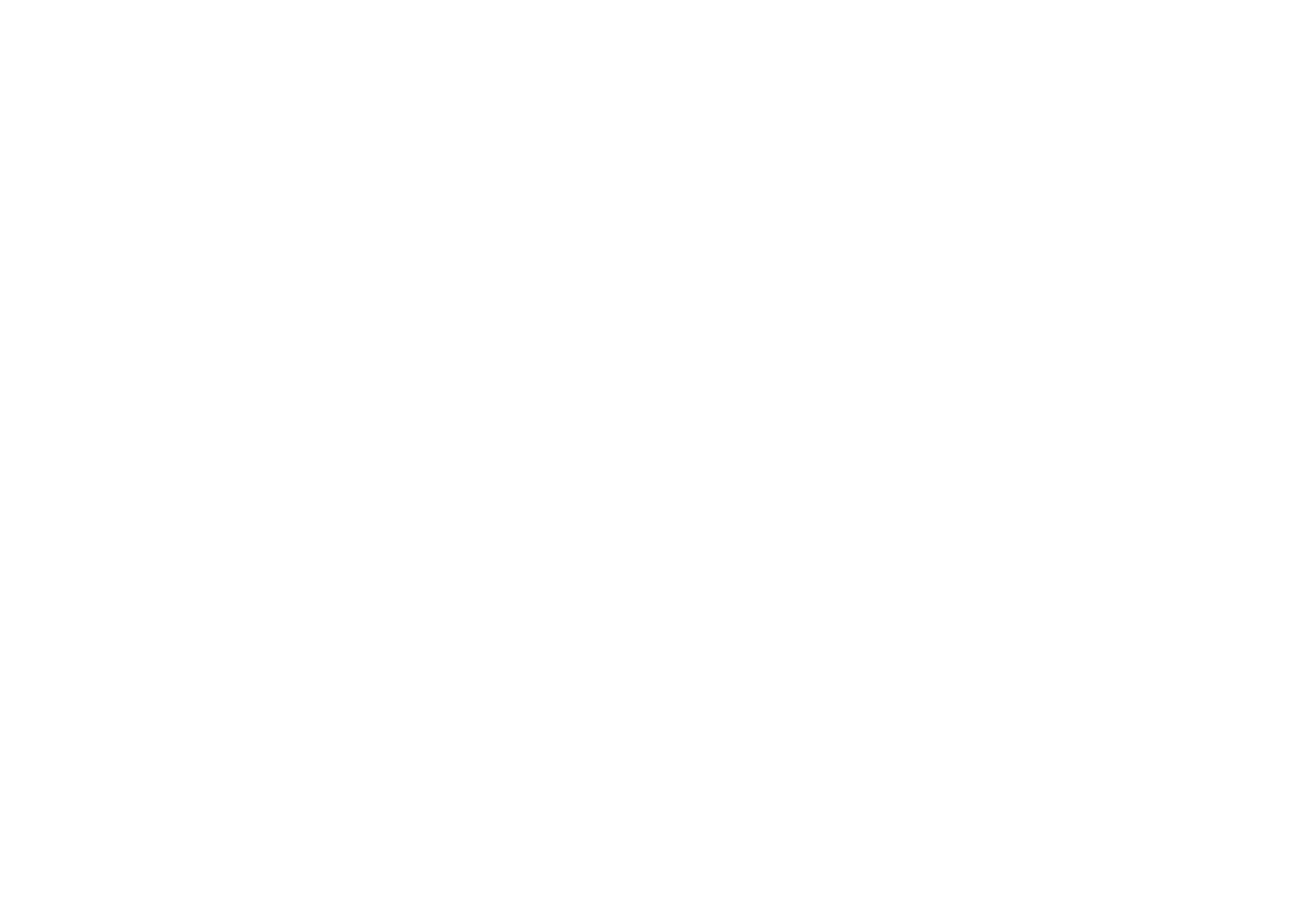
Creating Administrative Panels on WordPress Using PHP
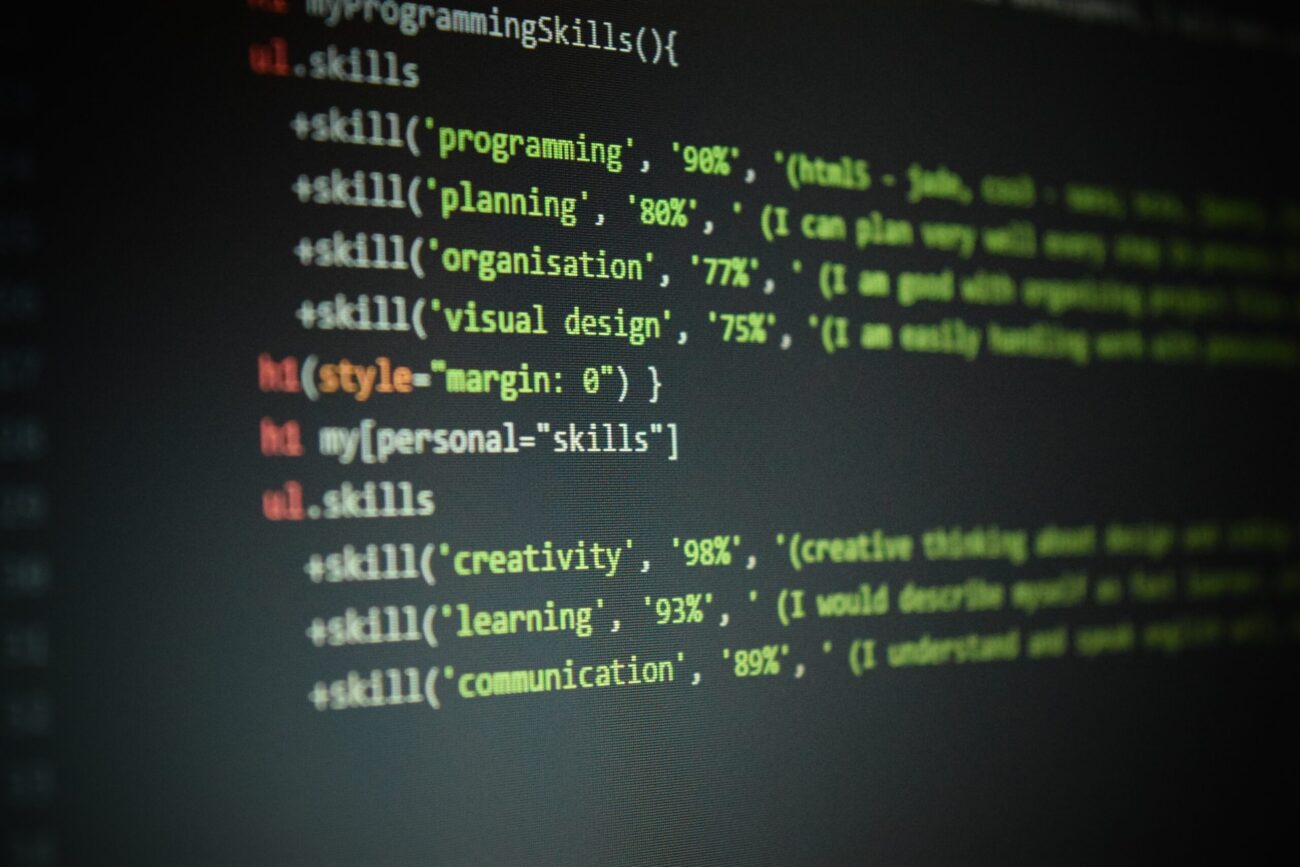
WordPress is a popular platform for creating and managing websites. One of the powerful tools it provides to developers is the ability to create custom administrative panels. In this article, we will explore how to use the PHP programming language to create custom administrative panels for managing your site’s content and settings.
Step 1: Getting Started
Before we begin, make sure you have WordPress installed and have basic knowledge of PHP.
Step 2: Creating a Plugin
To start, let’s create a new plugin that will contain our administrative panel. Create a new directory in the wp-content/plugins
folder and name it, for example, custom-admin-panel
.
Step 3: Main Plugin File
Inside the custom-admin-panel
directory, create a file named custom-admin-panel.php
. This file will be the main file of your plugin.
<?php
/*
Plugin Name: Custom Admin Panel
Description: Create a custom administrative panel for site management.
Version: 1.0
Author: Your Name
*/
// Your plugin code will go here
?>
Step 4: Adding the Administrative Panel
Next, let’s add a custom administrative panel to the WordPress admin page.
function custom_admin_panel_menu() {
add_menu_page(
'Custom Admin Panel', // Page title
'Custom Panel', // Menu title
'manage_options', // Role that has access to the page
'custom-admin-panel', // Unique page identifier
'custom_admin_panel_page', // Function to display content
'dashicons-admin-generic', // Menu icon
20 // Menu position
);
}
add_action('admin_menu', 'custom_admin_panel_menu');
Step 5: Creating the Panel Page
Now, let’s create a function to display the content of our administrative panel.
function custom_admin_panel_page() {
?>
<div class="wrap">
<h2>Custom Administrative Panel</h2>
<!-- Your HTML and forms will go here -->
</div>
<?php
}
Step 6: Adding Functionality
Let’s add an example of functionality, such as a form for changing settings.
function custom_admin_panel_page() {
?>
<div class="wrap">
<h2>Custom Administrative Panel</h2>
<form method="post" action="">
<?php wp_nonce_field('custom_admin_panel_nonce', 'custom_admin_panel_nonce'); ?>
<table class="form-table">
<tr valign="top">
<th scope="row">Setting 1</th>
<td><input type="text" name="setting_1" value="<?php echo esc_attr(get_option('setting_1')); ?>" /></td>
</tr>
<!-- Add additional settings here -->
</table>
<p class="submit"><input type="submit" class="button-primary" value="Save Changes" /></p>
</form>
</div>
<?php
}
Step 7: Handling Form Data
Add a function to handle form data and save settings.
function custom_admin_panel_save_settings() {
if (isset($_POST['custom_admin_panel_nonce']) && wp_verify_nonce($_POST['custom_admin_panel_nonce'], 'custom_admin_panel_nonce')) {
update_option('setting_1', sanitize_text_field($_POST['setting_1']));
// Handle other settings
}
}
add_action('admin_init', 'custom_admin_panel_save_settings');
Conclusion
In this article, we have covered how to create custom administrative panels on WordPress using the PHP programming language. We started by creating a plugin, added a menu item to the admin panel, created a panel page with a form for changing settings, and added a handler to save the data. You can now extend the functionality of your administrative panel by adding more fields and actions.
Please note that the examples provided in this article are basic demonstrations of the concept of creating administrative panels on WordPress using PHP. Your implementation may include additional design elements, security checks, and other details based on your needs.
Congratulations, you now have a basic understanding of how to create custom administrative panels on WordPress using PHP!