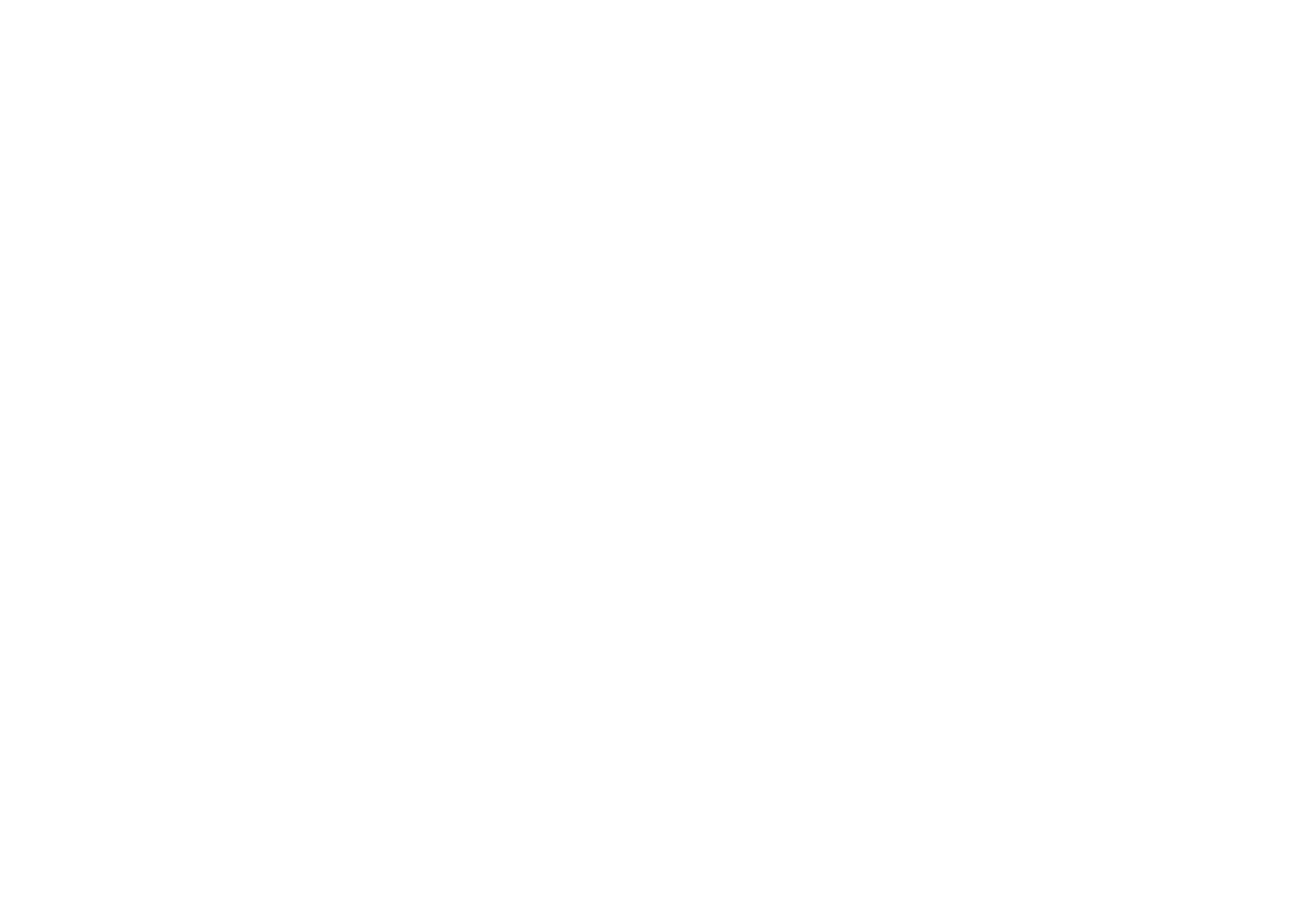
Adding and Displaying Various Types of Custom Fields to WordPress Posts Using PHP
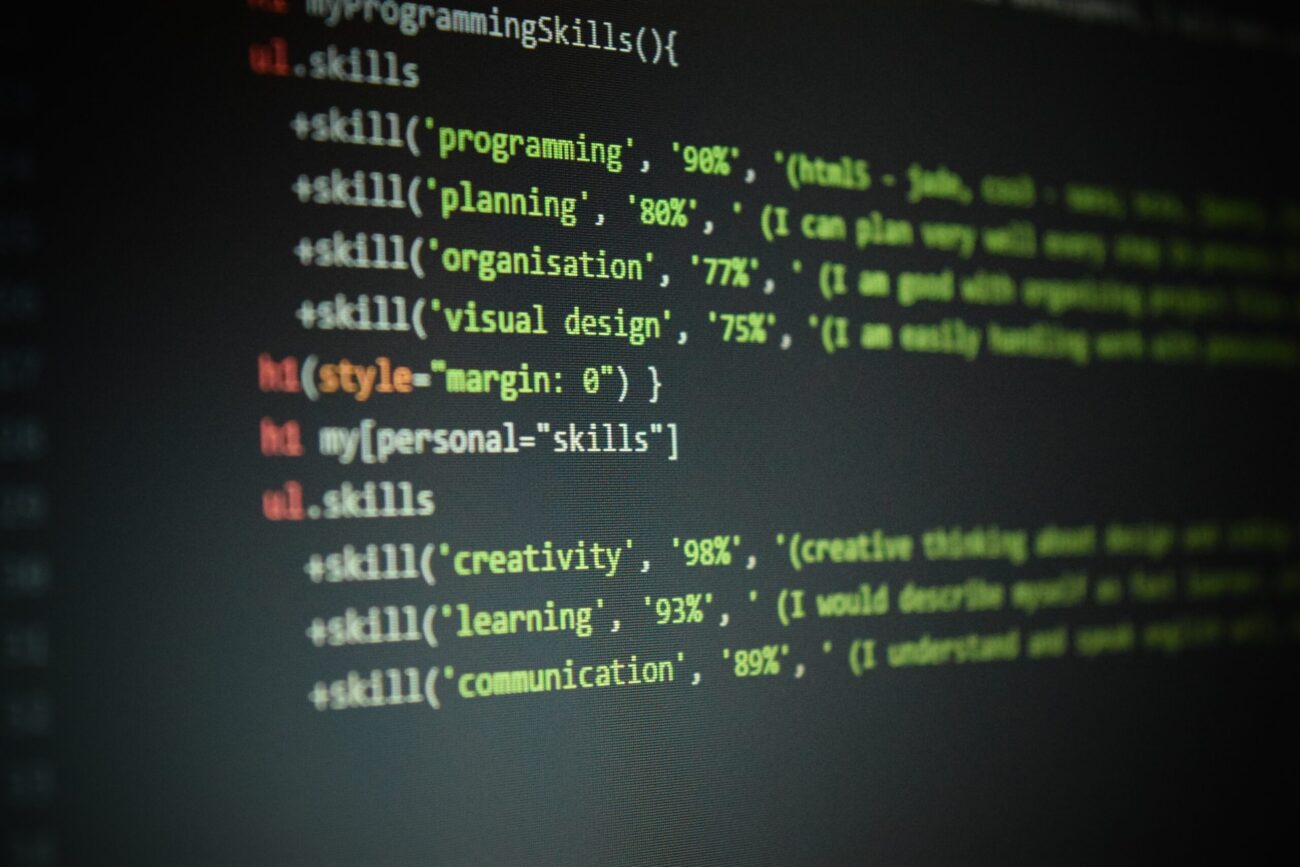
Creating interactive and customizable websites has become an essential part of modern web development. WordPress, as one of the most popular platforms for building websites and blogs, provides extensive capabilities for adding custom functionalities. In this article, we will explore how to create and add different types of custom fields to WordPress posts using PHP.
Understanding Custom Fields
Custom fields (or meta fields) are additional data that can be attached to posts, pages, users, or other post types in WordPress. They allow you to store and display additional information that is not included in the standard WordPress fields.
Examples of using custom fields could be:
- Adding ratings to articles.
- Displaying extra information about products on a shop page.
- Storing additional user details.
- Integrating with external APIs to show additional information.
Creating and Displaying Various Types of Custom Fields Using WordPress Functions
To add and display different types of custom fields, we will use WordPress functions that allow us to define, save, and display meta field data.
1. Text Field
Let’s imagine we are creating a cooking blog and need to add a field to specify the cooking time for each recipe.
Creating a Text Field:
function custom_cooking_time_field() {
$cooking_time = get_post_meta(get_the_ID(), 'cooking_time', true);
echo '<label for="cooking_time">Cooking Time (minutes): </label>';
echo '<input type="text" id="cooking_time" name="cooking_time" value="' . esc_attr($cooking_time) . '">';
}
add_action('add_meta_boxes', 'custom_cooking_time_field');
Saving and Displaying Text Field Data:
function save_custom_cooking_time_field($post_id) {
if (array_key_exists('cooking_time', $_POST)) {
update_post_meta(
$post_id,
'cooking_time',
sanitize_text_field($_POST['cooking_time'])
);
}
}
add_action('save_post', 'save_custom_cooking_time_field');
function display_custom_cooking_time_field($post) {
$cooking_time = get_post_meta($post->ID, 'cooking_time', true);
echo '<p><strong>Cooking Time:</strong> ' . esc_html($cooking_time) . ' minutes</p>';
}
add_action('admin_notices', 'display_custom_cooking_time_field');
2. Select Field
Let’s add a select field to specify the difficulty level of a recipe.
Creating a Select Field:
function custom_difficulty_level_field() {
$difficulty_levels = array(
'easy' => 'Easy',
'medium' => 'Medium',
'hard' => 'Hard',
);
$selected_level = get_post_meta(get_the_ID(), 'difficulty_level', true);
echo '<label for="difficulty_level">Difficulty Level: </label>';
echo '<select id="difficulty_level" name="difficulty_level">';
foreach ($difficulty_levels as $value => $label) {
echo '<option value="' . esc_attr($value) . '" ' . selected($selected_level, $value, false) . '>' . esc_html($label) . '</option>';
}
echo '</select>';
}
add_action('add_meta_boxes', 'custom_difficulty_level_field');
Saving and Displaying Select Field Data:
function save_custom_difficulty_level_field($post_id) {
if (array_key_exists('difficulty_level', $_POST)) {
update_post_meta(
$post_id,
'difficulty_level',
sanitize_text_field($_POST['difficulty_level'])
);
}
}
add_action('save_post', 'save_custom_difficulty_level_field');
function display_custom_difficulty_level_field($post) {
$difficulty_level = get_post_meta($post->ID, 'difficulty_level', true);
echo '<p><strong>Difficulty Level:</strong> ' . esc_html($difficulty_level) . '</p>';
}
add_action('admin_notices', 'display_custom_difficulty_level_field');
3. Radio Field
Let’s add a radio field to specify the meal type of a recipe.
Creating a Radio Field:
function custom_meal_type_field() {
$meal_types = array(
'breakfast' => 'Breakfast',
'lunch' => 'Lunch',
'dinner' => 'Dinner',
);
$selected_type = get_post_meta(get_the_ID(), 'meal_type', true);
echo '<label>Meal Type: </label><br>';
foreach ($meal_types as $value => $label) {
echo '<label>';
echo '<input type="radio" name="meal_type" value="' . esc_attr($value) . '" ' . checked($selected_type, $value, false) . '>';
echo esc_html($label);
echo '</label><br>';
}
}
add_action('add_meta_boxes', 'custom_meal_type_field');
Saving and Displaying Radio Field Data:
function save_custom_meal_type_field($post_id) {
if (array_key_exists('meal_type', $_POST)) {
update_post_meta(
$post_id,
'meal_type',
sanitize_text_field($_POST['meal_type'])
);
}
}
add_action('save_post', 'save_custom_meal_type_field');
function display_custom_meal_type_field($post) {
$meal_type = get_post_meta($post->ID, 'meal_type', true);
echo '<p><strong>Meal Type:</strong> ' . esc_html($meal_type) . '</p>';
}
add_action('admin_notices', 'display_custom_meal_type_field');
4. Checkbox Field
Let’s add a checkbox field to specify dietary preferences for a recipe.
Creating a Checkbox Field:
function custom_diets_checkbox_field() {
$diets = array(
'vegan' => 'Vegan',
'gluten_free' => 'Gluten-Free',
'paleo' => 'Paleo',
);
$selected_diets = get_post_meta(get_the_ID(), 'selected_diets', false);
echo '<label>Diets: </label><br>';
foreach ($diets as $value => $label) {
echo '<label>';
echo '<input type="checkbox" name="selected_diets[]" value="' . esc_attr($value) . '" ' . checked(in_array($value, $selected_diets), true, false) . '>';
echo esc_html($label);
echo '</label><br>';
}
}
add_action('add_meta_boxes', 'custom_diets_checkbox_field');
Saving and Displaying Checkbox Field Data:
function save_custom_diets_checkbox_field($post_id) {
if (array_key_exists('selected_diets', $_POST)) {
update_post_meta(
$post_id,
'selected_diets',
array_map('sanitize_text_field', $_POST['selected_diets'])
);
}
}
add_action('save_post', 'save_custom_diets_checkbox_field');
function display_custom_diets_checkbox_field($post) {
$selected_diets = get_post_meta($post->ID, 'selected_diets', false);
if (!empty($selected_diets)) {
echo '<p><strong>Diets:</strong> ' . implode(', ', array_map('esc_html', $selected_diets)) . '</p>';
}
}
add_action('admin_notices', 'display_custom_diets_checkbox_field');
5. Textarea Field
Let’s add a textarea field to provide recipe instructions.
Creating a Textarea Field:
function custom_recipe_instructions_field() {
$recipe_instructions = get_post_meta(get_the_ID(), 'recipe_instructions', true);
echo '<label for="recipe_instructions">Recipe Instructions: </label>';
echo '<textarea id="recipe_instructions" name="recipe_instructions">' . esc_textarea($recipe_instructions) . '</textarea>';
}
add_action('add_meta_boxes', 'custom_recipe_instructions_field');
Saving and Displaying Textarea Field Data:
function save_custom_recipe_instructions_field($post_id) {
if (array_key_exists('recipe_instructions', $_POST)) {
update_post_meta(
$post_id,
'recipe_instructions',
sanitize_textarea_field($_POST['recipe_instructions'])
);
}
}
add_action('save_post', 'save_custom_recipe_instructions_field');
function display_custom_recipe_instructions_field($post) {
$recipe_instructions = get_post_meta($post->ID, 'recipe_instructions', true);
echo '<p><strong>Recipe Instructions:</strong><br>' . esc_html($recipe_instructions) . '</p>';
}
add_action('admin_notices', 'display_custom_recipe_instructions_field');
6. Image Field
Let’s add an image field to provide a visual representation of the recipe.
Creating an Image Field:
function custom_recipe_image_field() {
$recipe_image = get_post_meta(get_the_ID(), 'recipe_image', true);
echo '<label for="recipe_image">Recipe Image: </label>';
echo '<input type="text" id="recipe_image" name="recipe_image" value="' . esc_attr($recipe_image) . '">';
echo '<input type="button" id="upload_recipe_image" class="button" value="Upload">';
}
add_action('add_meta_boxes', 'custom_recipe_image_field');
Saving and Displaying Image Field Data:
function save_custom_recipe_image_field($post_id) {
if (array_key_exists('recipe_image', $_POST)) {
update_post_meta(
$post_id,
'recipe_image',
sanitize_text_field($_POST['recipe_image'])
);
}
}
add_action('save_post', 'save_custom_recipe_image_field');
function display_custom_recipe_image_field($post) {
$recipe_image = get_post_meta($post->ID, 'recipe_image', true);
echo '<p><strong>Recipe Image:</strong><br><img src="' . esc_url($recipe_image) . '" alt="Recipe Image"></p>';
}
add_action('admin_notices', 'display_custom_recipe_image_field');
7. Image Gallery
Let’s add an image gallery field to showcase multiple images for a recipe.
Creating an Image Gallery Field:
function custom_recipe_gallery_field() {
$recipe_gallery = get_post_meta(get_the_ID(), 'recipe_gallery', true);
echo '<label for="recipe_gallery">Recipe Image Gallery: </label>';
echo '<input type="text" id="recipe_gallery" name="recipe_gallery" value="' . esc_attr($recipe_gallery) . '">';
echo '<input type="button" id="upload_recipe_gallery" class="button" value="Upload">';
}
add_action('add_meta_boxes', 'custom_recipe_gallery_field');
Saving and Displaying Image Gallery Field Data:
function save_custom_recipe_gallery_field($post_id) {
if (array_key_exists('recipe_gallery', $_POST)) {
update_post_meta(
$post_id,
'recipe_gallery',
sanitize_text_field($_POST['recipe_gallery'])
);
}
}
add_action('save_post', 'save_custom_recipe_gallery_field');
function display_custom_recipe_gallery_field($post) {
$recipe_gallery = get_post_meta($post->ID, 'recipe_gallery', true);
$images = explode(',', $recipe_gallery);
if (!empty($images)) {
echo '<p><strong>Recipe Image Gallery:</strong><br>';
foreach ($images as $image) {
echo '<img src="' . esc_url($image) . '" alt="Recipe Image">';
}
echo '</p>';
}
}
add_action('admin_notices', 'display_custom_recipe_gallery_field');
Advanced Capabilities
- Post Type Selection: You can choose which post types to add custom fields for. For example, you can limit them to posts, pages, or even your custom post types.
- Data Validation and Sanitization: Always validate and sanitize user inputs to prevent undesirable behavior and enhance security.
- Custom Field Interfaces: Consider using plugins that provide user-friendly interfaces for creating and managing custom fields without writing code.
Conclusion
Adding custom fields of various types can significantly extend your WordPress site’s functionality. You can create tailored elements and give users more options to interact with your content. By using WordPress functions and the provided examples, you’ll be able to successfully add and manage diverse custom fields on your site.