Essential Git Commands: A Comprehensive Guide
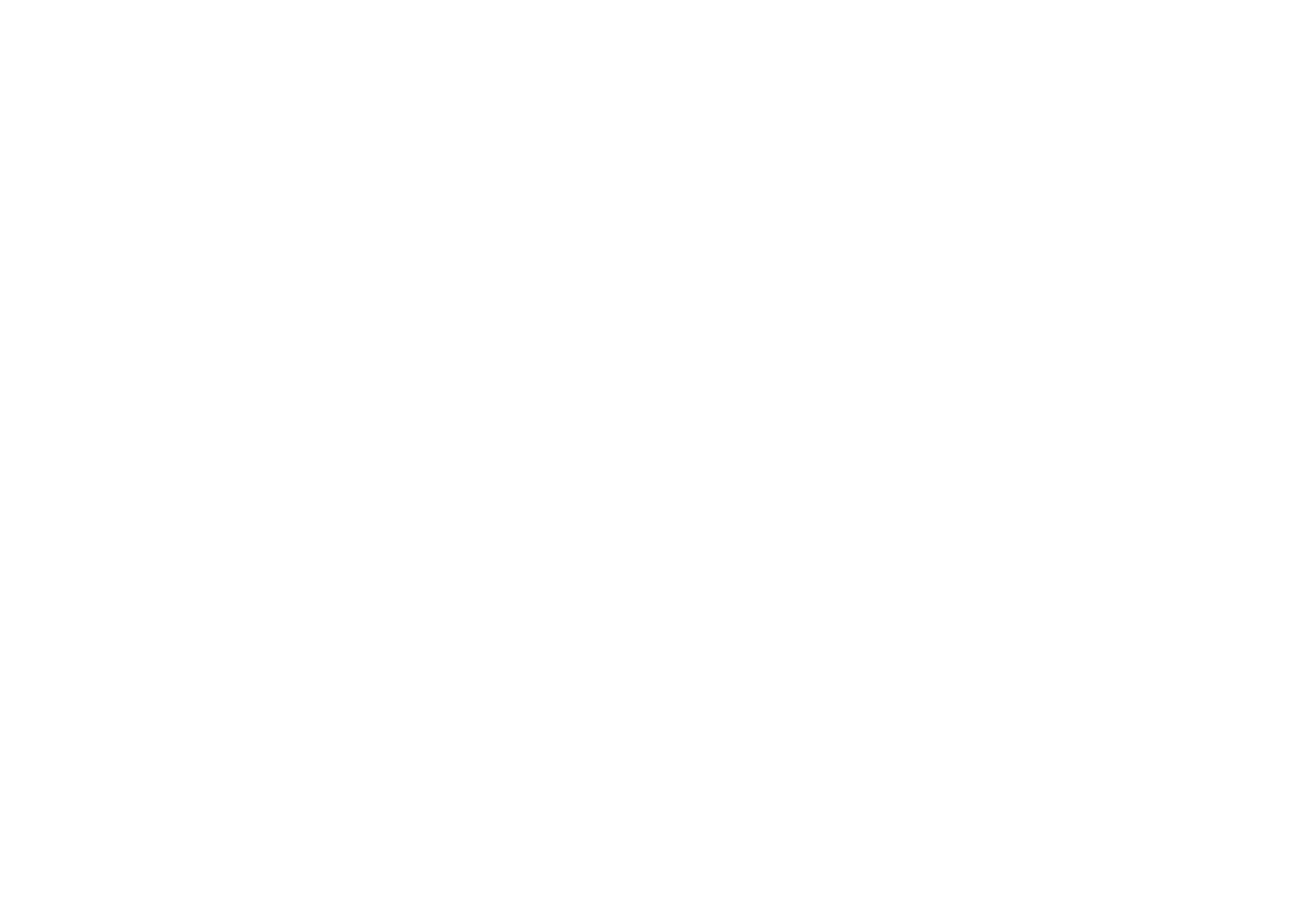
Git is a powerful version control system widely used by developers for effective management of codebases. Knowing key Git commands is essential for successful development and project management. Below is a list of the most important Git commands, detailed explanations of their functionality, and usage examples.
Setup and Configuration
git config
This command sets user parameters in Git, including user name and email, which is critical for authorship identification in your work. For example, setting your user name and email:
git config --global user.name "Your Name"
git config --global user.email "your_email@example.com"
Creating and Cloning Repositories
git init
Initializes a new local Git repository. This is the first step in creating a new project under Git management. In the project folder, just execute:
git init
git clone [url]
Cloning a repository means creating a local copy of an existing repository from a remote source. For example, cloning a GitHub repository:
git clone https://github.com/example/repo.git
Basics of Working with Branches
git branch
With git branch
, you can create, list, and delete branches. Creating a new branch for feature development:
git branch new-feature
git checkout
This command allows you to switch between branches or restore files in the working directory. To switch to the new-feature
branch:
git checkout new-feature
git merge [branch]
git merge
is used to merge changes from one branch into another. For instance, merging the new-feature
branch into the current branch:
git merge new-feature
Working with Changes
git status
git status
provides information about the status of files in the working directory and index — which files are modified but not added to the index, and which are ready to be committed:
git status
git add [file]
git add
adds file changes to the index, preparing them for commit. To add a specific file:
git add example.txt
git commit -m "[message]"
git commit
saves your changes in the repository. Each commit is accompanied by a message describing the made changes:
git commit -m "Added new functionality"
git diff
git diff
shows the difference between files in the working directory and index, helping to understand what changes have been made:
git diff
Remote Repositories
git push [remote] [branch]
Sending changes from a local repository to a remote repository is done through git push
. For example, to push changes to the master
branch on the origin
server:
git push origin master
git pull [remote]
git pull
fetches and integrates changes from a remote repository into a local one. It’s a combination of fetch
and merge
commands:
git pull origin master
git fetch [remote]
git fetch
downloads changes from a remote repository but does not integrate them into the current branch:
git fetch origin
Conclusion
These commands are the foundation for effective work with Git. Mastering them will allow you to better control the development process and collaborate with other developers. Regular use of Git will improve the quality and organization of your code.
An In-Depth Look at New CSS Features in 2022-2023
1. Container Queries
Container Queries enable styling elements based on their container’s dimensions, not just the viewport size, which is especially useful for adaptive design.
Example:
.container { container-type: inline-size; }
.card { container-name: container; background: lightblue; }
@container (min-width: 500px){ .card { background: pink; } }
In this example, .card
changes its background to pink if the .container
width exceeds 500 pixels.
2. :focus-visible
Pseudo-Class
:focus-visible
improves accessibility by adding focus indicators only when necessary, like for keyboard users.
Example:
button:focus-visible { outline: 2px solid blue; }
Here, the outline appears around the button only during keyboard navigation.
3. :has()
Selector
The :has()
selector allows for styling an element based on the presence of other elements inside it.
Example:
div:has(> p.highlight) { background-color: yellow; }
Here, div
gets a yellow background if it contains a child p
with the class highlight
.
4. Subgrid
Subgrid allows grid items to inherit grid layout from their parent grid.
Example:
.grid { display: grid; grid-template-columns: 1fr 1fr; }
.item { display: grid; grid-template-columns: subgrid; }
Inner elements .item
inherit the grid structure from their parent .grid
.
5. Accent-Color
The accent-color
property simplifies styling form control elements.
Example:
input[type="checkbox"] { accent-color: blue; }
This changes the checkbox color to blue.
6. Individual Transform Properties
These properties (scale, translate, rotate) simplify applying and modifying CSS transformations.
Example:
div { rotate: 45deg; scale: 1.5; }
Here, the div
is rotated by 45 degrees and scaled up by 1.5 times.
7. Wide-Gamut Color Spaces
These color spaces allow for the use of more saturated and vivid colors.
Example:
div { background-color: color(display-p3 1 0 0); }
This code sets a bright red color for div
in the display-p3
color space.
8. New Viewport Units
New units (svh
, svw
, lvh
, lvw
, dvh
, dvw
) provide more precise sizing of elements relative to the viewport size.
Example:
.large-element { height: 50lvh; /* Height for large viewports */ }
.small-element { width: 80svw; /* Width for small viewports */ }
.large-element
has a height of 50% of large viewports, and .small-element
has a width of 80% of small viewports.
9. Text-Wrap Balance
Text-wrap balance
improves text distribution in headings and titles.
Example:
h1 { text-wrap: balance; }
This evenly distributes the text in h1
headings, enhancing readability.
10. Initial-Letter
The initial-letter
property allows for custom styling of the first letter in a paragraph.
Example:
p::first-letter { initial-letter: 3 2; }
This sets the first letter of a paragraph p
to be the size of three lines and raised by two lines.
These innovations in CSS significantly expand the capabilities of web developers, making websites more adaptive, accessible, and visually appealing.
Creating interactive and customizable websites has become an essential part of modern web development. WordPress, as one of the most popular platforms for building websites and blogs, provides extensive capabilities for adding custom functionalities. In this article, we will explore how to create and add different types of custom fields to WordPress posts using PHP.
Understanding Custom Fields
Custom fields (or meta fields) are additional data that can be attached to posts, pages, users, or other post types in WordPress. They allow you to store and display additional information that is not included in the standard WordPress fields.
Examples of using custom fields could be:
- Adding ratings to articles.
- Displaying extra information about products on a shop page.
- Storing additional user details.
- Integrating with external APIs to show additional information.
Creating and Displaying Various Types of Custom Fields Using WordPress Functions
To add and display different types of custom fields, we will use WordPress functions that allow us to define, save, and display meta field data.
1. Text Field
Let’s imagine we are creating a cooking blog and need to add a field to specify the cooking time for each recipe.
Creating a Text Field:
function custom_cooking_time_field() {
$cooking_time = get_post_meta(get_the_ID(), 'cooking_time', true);
echo '<label for="cooking_time">Cooking Time (minutes): </label>';
echo '<input type="text" id="cooking_time" name="cooking_time" value="' . esc_attr($cooking_time) . '">';
}
add_action('add_meta_boxes', 'custom_cooking_time_field');
Saving and Displaying Text Field Data:
function save_custom_cooking_time_field($post_id) {
if (array_key_exists('cooking_time', $_POST)) {
update_post_meta(
$post_id,
'cooking_time',
sanitize_text_field($_POST['cooking_time'])
);
}
}
add_action('save_post', 'save_custom_cooking_time_field');
function display_custom_cooking_time_field($post) {
$cooking_time = get_post_meta($post->ID, 'cooking_time', true);
echo '<p><strong>Cooking Time:</strong> ' . esc_html($cooking_time) . ' minutes</p>';
}
add_action('admin_notices', 'display_custom_cooking_time_field');
2. Select Field
Let’s add a select field to specify the difficulty level of a recipe.
Creating a Select Field:
function custom_difficulty_level_field() {
$difficulty_levels = array(
'easy' => 'Easy',
'medium' => 'Medium',
'hard' => 'Hard',
);
$selected_level = get_post_meta(get_the_ID(), 'difficulty_level', true);
echo '<label for="difficulty_level">Difficulty Level: </label>';
echo '<select id="difficulty_level" name="difficulty_level">';
foreach ($difficulty_levels as $value => $label) {
echo '<option value="' . esc_attr($value) . '" ' . selected($selected_level, $value, false) . '>' . esc_html($label) . '</option>';
}
echo '</select>';
}
add_action('add_meta_boxes', 'custom_difficulty_level_field');
Saving and Displaying Select Field Data:
function save_custom_difficulty_level_field($post_id) {
if (array_key_exists('difficulty_level', $_POST)) {
update_post_meta(
$post_id,
'difficulty_level',
sanitize_text_field($_POST['difficulty_level'])
);
}
}
add_action('save_post', 'save_custom_difficulty_level_field');
function display_custom_difficulty_level_field($post) {
$difficulty_level = get_post_meta($post->ID, 'difficulty_level', true);
echo '<p><strong>Difficulty Level:</strong> ' . esc_html($difficulty_level) . '</p>';
}
add_action('admin_notices', 'display_custom_difficulty_level_field');
3. Radio Field
Let’s add a radio field to specify the meal type of a recipe.
Creating a Radio Field:
function custom_meal_type_field() {
$meal_types = array(
'breakfast' => 'Breakfast',
'lunch' => 'Lunch',
'dinner' => 'Dinner',
);
$selected_type = get_post_meta(get_the_ID(), 'meal_type', true);
echo '<label>Meal Type: </label><br>';
foreach ($meal_types as $value => $label) {
echo '<label>';
echo '<input type="radio" name="meal_type" value="' . esc_attr($value) . '" ' . checked($selected_type, $value, false) . '>';
echo esc_html($label);
echo '</label><br>';
}
}
add_action('add_meta_boxes', 'custom_meal_type_field');
Saving and Displaying Radio Field Data:
function save_custom_meal_type_field($post_id) {
if (array_key_exists('meal_type', $_POST)) {
update_post_meta(
$post_id,
'meal_type',
sanitize_text_field($_POST['meal_type'])
);
}
}
add_action('save_post', 'save_custom_meal_type_field');
function display_custom_meal_type_field($post) {
$meal_type = get_post_meta($post->ID, 'meal_type', true);
echo '<p><strong>Meal Type:</strong> ' . esc_html($meal_type) . '</p>';
}
add_action('admin_notices', 'display_custom_meal_type_field');
4. Checkbox Field
Let’s add a checkbox field to specify dietary preferences for a recipe.
Creating a Checkbox Field:
function custom_diets_checkbox_field() {
$diets = array(
'vegan' => 'Vegan',
'gluten_free' => 'Gluten-Free',
'paleo' => 'Paleo',
);
$selected_diets = get_post_meta(get_the_ID(), 'selected_diets', false);
echo '<label>Diets: </label><br>';
foreach ($diets as $value => $label) {
echo '<label>';
echo '<input type="checkbox" name="selected_diets[]" value="' . esc_attr($value) . '" ' . checked(in_array($value, $selected_diets), true, false) . '>';
echo esc_html($label);
echo '</label><br>';
}
}
add_action('add_meta_boxes', 'custom_diets_checkbox_field');
Saving and Displaying Checkbox Field Data:
function save_custom_diets_checkbox_field($post_id) {
if (array_key_exists('selected_diets', $_POST)) {
update_post_meta(
$post_id,
'selected_diets',
array_map('sanitize_text_field', $_POST['selected_diets'])
);
}
}
add_action('save_post', 'save_custom_diets_checkbox_field');
function display_custom_diets_checkbox_field($post) {
$selected_diets = get_post_meta($post->ID, 'selected_diets', false);
if (!empty($selected_diets)) {
echo '<p><strong>Diets:</strong> ' . implode(', ', array_map('esc_html', $selected_diets)) . '</p>';
}
}
add_action('admin_notices', 'display_custom_diets_checkbox_field');
5. Textarea Field
Let’s add a textarea field to provide recipe instructions.
Creating a Textarea Field:
function custom_recipe_instructions_field() {
$recipe_instructions = get_post_meta(get_the_ID(), 'recipe_instructions', true);
echo '<label for="recipe_instructions">Recipe Instructions: </label>';
echo '<textarea id="recipe_instructions" name="recipe_instructions">' . esc_textarea($recipe_instructions) . '</textarea>';
}
add_action('add_meta_boxes', 'custom_recipe_instructions_field');
Saving and Displaying Textarea Field Data:
function save_custom_recipe_instructions_field($post_id) {
if (array_key_exists('recipe_instructions', $_POST)) {
update_post_meta(
$post_id,
'recipe_instructions',
sanitize_textarea_field($_POST['recipe_instructions'])
);
}
}
add_action('save_post', 'save_custom_recipe_instructions_field');
function display_custom_recipe_instructions_field($post) {
$recipe_instructions = get_post_meta($post->ID, 'recipe_instructions', true);
echo '<p><strong>Recipe Instructions:</strong><br>' . esc_html($recipe_instructions) . '</p>';
}
add_action('admin_notices', 'display_custom_recipe_instructions_field');
6. Image Field
Let’s add an image field to provide a visual representation of the recipe.
Creating an Image Field:
function custom_recipe_image_field() {
$recipe_image = get_post_meta(get_the_ID(), 'recipe_image', true);
echo '<label for="recipe_image">Recipe Image: </label>';
echo '<input type="text" id="recipe_image" name="recipe_image" value="' . esc_attr($recipe_image) . '">';
echo '<input type="button" id="upload_recipe_image" class="button" value="Upload">';
}
add_action('add_meta_boxes', 'custom_recipe_image_field');
Saving and Displaying Image Field Data:
function save_custom_recipe_image_field($post_id) {
if (array_key_exists('recipe_image', $_POST)) {
update_post_meta(
$post_id,
'recipe_image',
sanitize_text_field($_POST['recipe_image'])
);
}
}
add_action('save_post', 'save_custom_recipe_image_field');
function display_custom_recipe_image_field($post) {
$recipe_image = get_post_meta($post->ID, 'recipe_image', true);
echo '<p><strong>Recipe Image:</strong><br><img src="' . esc_url($recipe_image) . '" alt="Recipe Image"></p>';
}
add_action('admin_notices', 'display_custom_recipe_image_field');
7. Image Gallery
Let’s add an image gallery field to showcase multiple images for a recipe.
Creating an Image Gallery Field:
function custom_recipe_gallery_field() {
$recipe_gallery = get_post_meta(get_the_ID(), 'recipe_gallery', true);
echo '<label for="recipe_gallery">Recipe Image Gallery: </label>';
echo '<input type="text" id="recipe_gallery" name="recipe_gallery" value="' . esc_attr($recipe_gallery) . '">';
echo '<input type="button" id="upload_recipe_gallery" class="button" value="Upload">';
}
add_action('add_meta_boxes', 'custom_recipe_gallery_field');
Saving and Displaying Image Gallery Field Data:
function save_custom_recipe_gallery_field($post_id) {
if (array_key_exists('recipe_gallery', $_POST)) {
update_post_meta(
$post_id,
'recipe_gallery',
sanitize_text_field($_POST['recipe_gallery'])
);
}
}
add_action('save_post', 'save_custom_recipe_gallery_field');
function display_custom_recipe_gallery_field($post) {
$recipe_gallery = get_post_meta($post->ID, 'recipe_gallery', true);
$images = explode(',', $recipe_gallery);
if (!empty($images)) {
echo '<p><strong>Recipe Image Gallery:</strong><br>';
foreach ($images as $image) {
echo '<img src="' . esc_url($image) . '" alt="Recipe Image">';
}
echo '</p>';
}
}
add_action('admin_notices', 'display_custom_recipe_gallery_field');
Advanced Capabilities
- Post Type Selection: You can choose which post types to add custom fields for. For example, you can limit them to posts, pages, or even your custom post types.
- Data Validation and Sanitization: Always validate and sanitize user inputs to prevent undesirable behavior and enhance security.
- Custom Field Interfaces: Consider using plugins that provide user-friendly interfaces for creating and managing custom fields without writing code.
Conclusion
Adding custom fields of various types can significantly extend your WordPress site’s functionality. You can create tailored elements and give users more options to interact with your content. By using WordPress functions and the provided examples, you’ll be able to successfully add and manage diverse custom fields on your site.
Websites built on the WordPress and PHP platforms remain popular among web developers due to their flexibility and customization options. However, successful web development involves not only design and functionality but also search engine optimization (SEO). In this article, we will explore key steps for optimizing SEO for WordPress and PHP websites, along with providing code examples for better understanding of the process.
Table of Contents:
- Choosing the Right Keywords
- Content Optimization
- URL Structure
- Improving Loading Speed
- Utilizing Microdata
- Code Examples
- Conclusion
1. Choosing the Right Keywords
Selecting keywords is one of the most crucial aspects of SEO optimization. Identify keywords that best describe the content of your website. Research the popularity and competition of these keywords using tools like Google Keyword Planner.
Example code for keyword identification in WordPress:
// Get meta tags and keywords for the current page
$meta_tags = get_post_meta( get_the_ID(), '_yoast_wpseo_metakeywords', true );
$keywords = explode( ',', $meta_tags );
// Display keywords in the template
echo '<ul>';
foreach ( $keywords as $keyword ) {
echo '<li>' . trim( $keyword ) . '</li>';
}
echo '</ul>';
2. Content Optimization
Content plays a crucial role in SEO optimization. Create unique, valuable, and informative content for users. Include keywords in headings, subheadings, and paragraphs, but do so naturally and organically.
Example code for content optimization in PHP:
// Get page content
$content = get_the_content();
// Replace keywords with links within the content
$target_keyword = 'SEO optimization';
$linked_keyword = '<a href="/blog/seo-tips/">SEO optimization</a>';
$optimized_content = str_replace( $target_keyword, $linked_keyword, $content );
// Display optimized content
echo $optimized_content;
3. URL Structure
URL structure plays a role in both user-friendliness and search engine optimization. Use human-readable URLs containing keywords and avoid lengthy and cryptic parameters.
Example code for URL structure customization in WordPress:
// Modify URL structure settings
function custom_permalink_structure() {
global $wp_rewrite;
$wp_rewrite->set_permalink_structure( '/%category%/%postname%/' );
$wp_rewrite->flush_rules();
}
add_action( 'init', 'custom_permalink_structure' );
4. Improving Loading Speed
Page loading speed affects search engine rankings and user experience. Optimize images, minimize CSS and JavaScript, utilize caching, and use Content Delivery Networks (CDNs).
Example code for image loading optimization in PHP:
// Get a list of images on the page
$images = get_attached_media( 'image' );
foreach ( $images as $image ) {
$image_url = wp_get_attachment_image_url( $image->ID, 'full' );
echo '<img src="' . $image_url . '" alt="' . get_post_meta( $image->ID, '_wp_attachment_image_alt', true ) . '">';
}
5. Utilizing Microdata
Microdata helps search engines understand your website’s content and display additional information in search results. Use structured data to mark up information such as organizations, recipes, reviews, and more.
Example code for adding microdata to a page in WordPress:
// Add microdata for an organization
function add_organization_schema() {
$schema = array(
'@context' => 'http://schema.org',
'@type' => 'Organization',
'name' => 'Your Company Name',
'url' => 'https://www.example.com',
);
echo '<script type="application/ld+json">' . json_encode( $schema ) . '</script>';
}
add_action( 'wp_head', 'add_organization_schema' );
6. Code Examples
In this section of the article, we’ve covered only a small set of code examples for SEO optimization on WordPress and PHP websites. For a comprehensive optimization approach, it’s recommended to explore additional methods and strategies, as well as stay updated with the latest SEO trends.
7. Conclusion
SEO optimization for WordPress and PHP websites is a crucial step in development that helps improve a site’s visibility in search engines and attract more organic traffic. In this article, we’ve covered the fundamental optimization steps, including keyword selection, content optimization, URL structure, improving loading speed, and utilizing microdata.
Remember that SEO is an ongoing process that requires continuous updates and adaptations. Stay informed about new trends, test different approaches, and strive to create high-quality, valuable content for your users.
WordPress is a popular platform for creating and managing websites. One of the powerful tools it provides to developers is the ability to create custom administrative panels. In this article, we will explore how to use the PHP programming language to create custom administrative panels for managing your site’s content and settings.
Step 1: Getting Started
Before we begin, make sure you have WordPress installed and have basic knowledge of PHP.
Step 2: Creating a Plugin
To start, let’s create a new plugin that will contain our administrative panel. Create a new directory in the wp-content/plugins
folder and name it, for example, custom-admin-panel
.
Step 3: Main Plugin File
Inside the custom-admin-panel
directory, create a file named custom-admin-panel.php
. This file will be the main file of your plugin.
<?php
/*
Plugin Name: Custom Admin Panel
Description: Create a custom administrative panel for site management.
Version: 1.0
Author: Your Name
*/
// Your plugin code will go here
?>
Step 4: Adding the Administrative Panel
Next, let’s add a custom administrative panel to the WordPress admin page.
function custom_admin_panel_menu() {
add_menu_page(
'Custom Admin Panel', // Page title
'Custom Panel', // Menu title
'manage_options', // Role that has access to the page
'custom-admin-panel', // Unique page identifier
'custom_admin_panel_page', // Function to display content
'dashicons-admin-generic', // Menu icon
20 // Menu position
);
}
add_action('admin_menu', 'custom_admin_panel_menu');
Step 5: Creating the Panel Page
Now, let’s create a function to display the content of our administrative panel.
function custom_admin_panel_page() {
?>
<div class="wrap">
<h2>Custom Administrative Panel</h2>
<!-- Your HTML and forms will go here -->
</div>
<?php
}
Step 6: Adding Functionality
Let’s add an example of functionality, such as a form for changing settings.
function custom_admin_panel_page() {
?>
<div class="wrap">
<h2>Custom Administrative Panel</h2>
<form method="post" action="">
<?php wp_nonce_field('custom_admin_panel_nonce', 'custom_admin_panel_nonce'); ?>
<table class="form-table">
<tr valign="top">
<th scope="row">Setting 1</th>
<td><input type="text" name="setting_1" value="<?php echo esc_attr(get_option('setting_1')); ?>" /></td>
</tr>
<!-- Add additional settings here -->
</table>
<p class="submit"><input type="submit" class="button-primary" value="Save Changes" /></p>
</form>
</div>
<?php
}
Step 7: Handling Form Data
Add a function to handle form data and save settings.
function custom_admin_panel_save_settings() {
if (isset($_POST['custom_admin_panel_nonce']) && wp_verify_nonce($_POST['custom_admin_panel_nonce'], 'custom_admin_panel_nonce')) {
update_option('setting_1', sanitize_text_field($_POST['setting_1']));
// Handle other settings
}
}
add_action('admin_init', 'custom_admin_panel_save_settings');
Conclusion
In this article, we have covered how to create custom administrative panels on WordPress using the PHP programming language. We started by creating a plugin, added a menu item to the admin panel, created a panel page with a form for changing settings, and added a handler to save the data. You can now extend the functionality of your administrative panel by adding more fields and actions.
Please note that the examples provided in this article are basic demonstrations of the concept of creating administrative panels on WordPress using PHP. Your implementation may include additional design elements, security checks, and other details based on your needs.
Congratulations, you now have a basic understanding of how to create custom administrative panels on WordPress using PHP!
An API (Application Programming Interface) is a set of instructions and protocols that allow different programs to interact with each other. In the case of WordPress, an API enables you to create, modify, and retrieve data from your website using other applications or services. In this article, we will explore how to work with APIs in WordPress and PHP to extend the functionality of your website.
Introduction to the WordPress API
WordPress provides several different APIs for interacting with your website. One of the key APIs is the REST API, which allows you to send and receive data from your site using standard HTTP methods such as GET, POST, PUT, and DELETE. The REST API opens up vast possibilities for integrating your site with other applications and services.
Authentication and Obtaining an API Key
Before you start using the REST API, you’ll need an API key. To do this, go to the WordPress admin panel and navigate to «Settings» -> «General». Scroll down and find the «REST API» section. Here, you can create and manage API keys.
After creating the key, remember to keep it secure as it will be used for authentication in your API requests.
Examples of Using the REST API
Let’s consider several examples of using the REST API in WordPress.
Getting a List of Posts
To retrieve a list of all posts from your site, you can make a GET request as follows:
$api_url = 'https://your_site/wp-json/wp/v2/posts';
$response = wp_remote_get( $api_url );
$data = wp_remote_retrieve_body( $response );
$posts = json_decode( $data );
Creating a New Post
To create a new post on your site, you can use a POST request:
$api_url = 'https://your_site/wp-json/wp/v2/posts';
$data = array(
'title' => 'New Post',
'content' => 'Content of the new post...',
'status' => 'publish'
);
$response = wp_remote_post( $api_url, array(
'headers' => array(
'Authorization' => 'Bearer your_api_key',
),
'body' => json_encode( $data )
));
Updating a Post
To update an existing post, you can use a PUT request:
$post_id = 123; // ID of the post to update
$api_url = 'https://your_site/wp-json/wp/v2/posts/' . $post_id;
$data = array(
'title' => 'New Post Title',
'content' => 'New content of the post...'
);
$response = wp_remote_request( $api_url, array(
'method' => 'PUT',
'headers' => array(
'Authorization' => 'Bearer your_api_key',
),
'body' => json_encode( $data )
));
Deleting a Post
To delete a post, use a DELETE request:
$post_id = 123; // ID of the post to delete
$api_url = 'https://your_site/wp-json/wp/v2/posts/' . $post_id;
$response = wp_remote_request( $api_url, array(
'method' => 'DELETE',
'headers' => array(
'Authorization' => 'Bearer your_api_key',
)
));
Using PHP for API Interaction
In addition to the WordPress REST API, PHP offers a multitude of built-in functions for working with external APIs. Let’s explore a few examples.
Sending a GET Request
To send a GET request to an external API, you can use the file_get_contents
function:
$api_url = 'https://api.example.com/data';
$response = file_get_contents( $api_url );
$data = json_decode( $response );
Sending a POST Request
For sending a POST request, you can utilize the curl
function:
$api_url = 'https://api.example.com/create';
$data = array(
'param1' => 'value1',
'param2' => 'value2'
);
$ch = curl_init( $api_url );
curl_setopt( $ch, CURLOPT_POST, 1 );
curl_setopt( $ch, CURLOPT_POSTFIELDS, http_build_query( $data ) );
curl_setopt( $ch, CURLOPT_RETURNTRANSFER, true );
$response = curl_exec( $ch );
curl_close( $ch );
Authenticating with an External API
If authentication is required for an external API, you can pass necessary headers:
$api_url = 'https://api.example.com/data';
$headers = array(
'Authorization: Bearer your_api_key',
'Content-Type: application/json'
);
$options = array(
'http' => array(
'header' => implode( "\r\n", $headers )
)
);
$context = stream_context_create( $options );
$response = file_get_contents( $api_url, false, $context );
$data = json_decode( $response );
Conclusion
Working with APIs in WordPress and PHP offers vast opportunities for extending your website’s functionality. You can retrieve data, create and update content, as well as integrate your site with other applications and services. Thanks to the convenience and versatility of APIs, your website can interact seamlessly with the digital ecosystem, providing a richer user experience and opening doors to innovative possibilities.
Security in web development is one of the key aspects that requires special attention when creating websites. In this article, we will explore important principles and methods for ensuring security in the development of websites on the WordPress platform using the PHP programming language. We will also provide code examples that will help you create reliable and secure web applications.
1. Software Updates
The WordPress platform and the PHP programming language are regularly updated by developers to address vulnerabilities and enhance security. Periodically check for available updates for your website and make sure you are using the latest versions of WordPress and PHP.
// Example of checking for WordPress updates
if (function_exists('wp_version_check')) {
$updates = wp_version_check();
if (!empty($updates) && $updates['response'] == 'upgrade') {
// Display a warning about available updates
}
}
2. Protection Against SQL Injections
SQL injections are a common method of attacking web applications, including WordPress websites. Use prepared statements and data escaping to prevent this vulnerability.
// Example of a prepared statement
global $wpdb;
$username = $_POST['username'];
$password = $_POST['password'];
$query = $wpdb->prepare("SELECT * FROM wp_users WHERE user_login = %s AND user_pass = %s", $username, $password);
$results = $wpdb->get_results($query);
3. Proper Handling of User Input
Always assume that user input can be dangerous and validate and filter data before using it.
// Example of email validation
$email = $_POST['email'];
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
// Actions for a valid email address
} else {
// Error handling
}
4. Protection Against Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery (CSRF) is another common type of attack. Use protection mechanisms such as generating and checking CSRF tokens.
// Example of generating and checking a CSRF token
session_start();
$csrf_token = bin2hex(random_bytes(32));
$_SESSION['csrf_token'] = $csrf_token;
// In the form
<input type="hidden" name="csrf_token" value="<?php echo $csrf_token; ?>">
// When processing the request
if ($_POST['csrf_token'] === $_SESSION['csrf_token']) {
// Actions for a valid CSRF token
} else {
// CSRF error handling
}
5. Limiting Access Rights
Do not grant unnecessary access rights to users and administrators. Use the principle of least privilege to restrict the capabilities of potential attackers.
// Example of limiting access rights
if (current_user_can('edit_posts')) {
// Actions for users with post editing rights
} else {
// Access limitation
}
6. Protection Against Malicious File Uploads
Ensure that files uploaded by users are checked for malicious code. Use whitelists of allowed file types and validate the contents of uploaded files.
// Example of checking the uploaded file type
$allowed_file_types = array('jpg', 'png', 'gif');
$uploaded_file = $_FILES['file'];
$file_extension = pathinfo($uploaded_file['name'], PATHINFO_EXTENSION);
if (in_array($file_extension, $allowed_file_types)) {
// Process the uploaded file
} else {
// File upload error
}
7. Protection of Confidential Data
Avoid storing confidential data, such as passwords, in plain text. Use hashing and salting to ensure the security of stored information.
// Example of password hashing
$password = $_POST['password'];
$salt = 'somerandomstring'; // Unique salt for each user
$hashed_password = hash('sha256', $password . $salt);
Conclusion
Ensuring security in WordPress and PHP website development is a complex but necessary task. Adhering to the principles mentioned above and using code examples will allow you to create reliable and secure web applications. Always stay updated on the latest security trends and continuously improve your knowledge to stay ahead of potential threats.
In the modern world of web development, interacting with databases has become an integral part of creating powerful and functional web applications. One of the most popular tools for developing dynamic websites and applications is PHP. When combined with the widely used content management platform WordPress, PHP offers developers a multitude of opportunities for integrating databases. In this article, we will explore methods of integrating databases into web applications using PHP and WordPress, provide code examples, and explain each step of the process in detail.
Part 1: Basics of Working with Databases
Before diving into integrating databases into PHP and WordPress, let’s understand the basics of working with databases. A database is a structured repository of information that can be efficiently managed and retrieved. To interact with a database, we will use the Structured Query Language (SQL).
Part 2: Creating and Configuring the Database
Before we can begin integrating the database into our web application, we need to create and configure the database. For our example, let’s consider creating a simple database to store user information for our web application. We will use MySQL, one of the most popular database management systems.
Code Example SQL:
CREATE DATABASE myapp_db;
USE myapp_db;
CREATE TABLE users (
id INT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) NOT NULL,
password VARCHAR(255) NOT NULL
);
Part 3: Connecting to the Database
Now that the database is created, let’s learn how to establish a connection to it from PHP code. We will use the PHP Data Objects (PDO) extension, which provides an abstraction layer for accessing different databases.
Code Example PHP:
$dsn = "mysql:host=localhost;dbname=myapp_db";
$username = "username";
$password = "password";
try {
$pdo = new PDO($dsn, $username, $password);
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "Connected to the database";
} catch (PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
Part 4: Executing Database Queries
After successfully connecting to the database, we can execute queries to add, update, and retrieve data. Let’s look at examples of executing different queries using PDO.
Code Example PHP:
// Inserting a new user
$query = "INSERT INTO users (username, email, password) VALUES (?, ?, ?)";
$stmt = $pdo->prepare($query);
$username = "newuser";
$email = "newuser@example.com";
$password = password_hash("password123", PASSWORD_DEFAULT);
$stmt->execute([$username, $email, $password]);
echo "New user added";
// Retrieving a list of users
$query = "SELECT * FROM users";
$stmt = $pdo->query($query);
while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) {
echo "ID: " . $row['id'] . ", Name: " . $row['username'] . ", Email: " . $row['email'] . "<br>";
}
Part 5: Integration with WordPress
Next, we’ll move on to integrating the database with WordPress, a popular content management platform. WordPress provides its own methods for working with databases, making interaction easier and ensuring data security.
Code Example:
global $wpdb;
// Inserting a new record into a WordPress table
$table_name = $wpdb->prefix . 'custom_table';
$data = array('name' => 'John', 'email' => 'john@example.com');
$wpdb->insert($table_name, $data);
echo "New record added";
// Retrieving data from a WordPress table
$results = $wpdb->get_results("SELECT * FROM $table_name");
foreach ($results as $result) {
echo "ID: " . $result->id . ", Name: " . $result->name . ", Email: " . $result->email . "<br>";
}
Conclusion
Integrating databases into web applications using PHP and WordPress is a key aspect of developing modern dynamic websites and applications. In this article, we have covered the basics of working with databases, creating and configuring a database, connecting to it from PHP code, executing queries, and integrating with WordPress. Mastering these concepts will enable you to develop powerful and efficient web applications capable of working with data and providing an enhanced user experience.
Creating plugins for WordPress using the PHP programming language is an essential part of extending the functionality of the WordPress platform. In this article, we will discuss key aspects of plugin development and provide you with a solid understanding of how to embark on this exciting process.
Part 1: Fundamentals of WordPress Plugin Development
1. Preparation for Development:
Before diving into plugin creation, it’s important to set up your development environment. Install WordPress on a local server or use an online development environment. Create a separate folder within the wp-content/plugins
directory for your plugin.
2. Creating a Simple Plugin:
Let’s start by creating a minimal plugin. In your plugin’s folder, create a file named my-plugin.php
and add basic information:
<?php
/*
Plugin Name: My Plugin
Description: Example simple plugin for WordPress.
Version: 1.0
Author: Your Name
*/
This establishes a starting point for your plugin.
Part 2: Extending Functionality
3. Adding a New Admin Menu Item:
Let’s add a new item to the WordPress admin menu. Use the following code:
function my_plugin_menu() {
add_menu_page(
'My Page',
'My Page',
'manage_options',
'my-plugin-slug',
'my_plugin_page'
);
}
function my_plugin_page() {
echo '<div class="wrap"><h2>My Page</h2><p>Hello, world!</p></div>';
}
add_action('admin_menu', 'my_plugin_menu');
Part 3: Interacting with the Database
4. Creating a Database Table:
Plugins often require data storage. Let’s create a table in the database:
function create_custom_table() {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_table';
$charset_collate = $wpdb->get_charset_collate();
$sql = "CREATE TABLE $table_name (
id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(100) NOT NULL,
email VARCHAR(100) NOT NULL,
PRIMARY KEY (id)
) $charset_collate;";
require_once(ABSPATH . 'wp-admin/includes/upgrade.php');
dbDelta($sql);
}
register_activation_hook(__FILE__, 'create_custom_table');
5. Interacting with the Database:
Let’s continue by adding functions for data insertion and retrieval:
function insert_data($name, $email) {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_table';
$wpdb->insert(
$table_name,
array(
'name' => $name,
'email' => $email,
)
);
}
function get_data() {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_table';
$results = $wpdb->get_results("SELECT * FROM $table_name");
return $results;
}
Part 4: Creating a Custom Widget
6. Creating a Custom Widget:
Plugins can also include custom widgets. Let’s create a widget that displays a list of «Products»:
class Product_Widget extends WP_Widget {
function __construct() {
parent::__construct(
'product_widget',
'Product Widget',
array('description' => 'Displays a list of products')
);
}
public function widget($args, $instance) {
echo $args['before_widget'];
echo $args['before_title'] . 'Products' . $args['after_title'];
$products = get_data();
echo '<ul>';
foreach ($products as $product) {
echo '<li>' . $product->name . '</li>';
}
echo '</ul>';
echo $args['after_widget'];
}
}
function register_product_widget() {
register_widget('Product_Widget');
}
add_action('widgets_init', 'register_product_widget');
Conclusion:
This article provided you with an introduction to WordPress plugin development using PHP. We covered the basics of creating a plugin, adding a new admin menu page, interacting with the database, and creating a custom widget. Plugin development is a powerful way to customize and extend your WordPress website’s functionality. Use this knowledge to create innovative solutions and enhance your projects.
WordPress content management system is a powerful tool for creating and managing websites. One of its key features is the ability to create custom themes, which allow you to have full control over the appearance and functionality of your website. In this article, we will provide a detailed guide on creating custom WordPress themes using the PHP programming language. We’ll cover all the steps, from initial setup to adding custom templates and functions.
Step 1: Preparing for Theme Development
Before diving into theme development, ensure that you have WordPress installed on your hosting. Create a folder for your theme within the «wp-content/themes» directory and give it a unique name.
Step 2: Creating Core Theme Files
Your theme should have at least two core files: «style.css» and «index.php». In «style.css,» you’ll define information about your theme, and «index.php» will serve as the template for the main page.
Example «style.css»:
/*
Theme Name: My Custom Theme
Author: Your Name
Description: Description of your theme
Version: 1.0
*/
/* Additional styles here */
Example «index.php»:
<?php get_header(); ?>
<main id="main-content">
<?php if (have_posts()) : while (have_posts()) : the_post(); ?>
<article <?php post_class(); ?>>
<h2><a href="<?php the_permalink(); ?>"><?php the_title(); ?></a></h2>
<div class="post-content"><?php the_content(); ?></div>
</article>
<?php endwhile; endif; ?>
</main>
<?php get_sidebar(); ?>
<?php get_footer(); ?>
Step 3: Adding Custom Templates
To make your theme more flexible, you can add custom templates for different types of content, such as archive pages, blog pages, etc.
Example «archive.php»:
<?php get_header(); ?>
<main id="main-content">
<h1>Archive</h1>
<?php if (have_posts()) : while (have_posts()) : the_post(); ?>
<article <?php post_class(); ?>>
<h2><a href="<?php the_permalink(); ?>"><?php the_title(); ?></a></h2>
<div class="post-content"><?php the_excerpt(); ?></div>
</article>
<?php endwhile; endif; ?>
</main>
<?php get_sidebar(); ?>
<?php get_footer(); ?>
Step 4: Adding Custom Functions
You can expand your theme’s functionality by adding custom functions to the «functions.php» file.
Example of adding a custom function:
function custom_theme_setup() {
add_theme_support('title-tag');
add_theme_support('post-thumbnails');
register_nav_menus(array(
'primary-menu' => 'Primary Menu',
));
}
add_action('after_setup_theme', 'custom_theme_setup');
Step 5: Theme Styling
Create a «css» folder within your theme and add a «style.css» file to define your theme’s styles. You can also use CSS preprocessors like Sass or LESS.
Conclusion:
Creating custom WordPress themes using PHP is an exciting process that empowers you to have full control over your website’s appearance and functionality. In this article, we’ve covered key steps, from setup and core file creation to adding custom templates and functions. Now you have an excellent guide to create unique custom WordPress themes using PHP. Don’t limit yourself—experiment, improve, and share your creative solutions with the world!
WordPress is one of the most popular platforms for creating websites and blogs, but sometimes its performance can leave something to be desired. With a constantly growing volume of content and features, performance optimization becomes a key task for webmasters and developers. In this article, we will explore how using PHP can help optimize your WordPress site’s performance. We will also provide code examples for a better understanding.
1. Caching with PHP
One of the ways to significantly improve WordPress performance is through caching. Caching allows you to store the results of queries and operations for later use, reducing server load and speeding up page loading.
Code Example:
// Enable page-level caching
if (!defined('WP_CACHE')) {
define('WP_CACHE', true);
}
// Use a cache object to store data
$cache_key = 'recent_posts';
$recent_posts = wp_cache_get($cache_key);
if (false === $recent_posts) {
$recent_posts = get_recent_posts_from_database();
wp_cache_set($cache_key, $recent_posts, 'posts', 300); // Cache for 5 minutes
}
// Use $recent_posts to display data on the page
2. Database Optimization
Efficient use of the database is another important aspect of performance optimization. PHP provides many tools for working with the database and improving its performance.
Code Example:
// Use prepared statements to prevent SQL injections
$user_id = $_GET['user_id'];
$prepared_query = $wpdb->prepare("SELECT * FROM {$wpdb->prefix}users WHERE ID = %d", $user_id);
$results = $wpdb->get_results($prepared_query);
// Use indexes to speed up queries
$wpdb->query("ALTER TABLE {$wpdb->prefix}posts ADD INDEX post_title_index (post_title)");
// Limit the number of retrieved records
$limit = 10;
$posts = $wpdb->get_results("SELECT * FROM {$wpdb->prefix}posts LIMIT $limit");
3. Query Optimization
Unnecessary and inefficient queries can slow down page loading. By using PHP, you can optimize queries and minimize server load.
Code Example:
// Select only necessary fields from the database
$posts = $wpdb->get_results("SELECT ID, post_title FROM {$wpdb->prefix}posts");
// Use caching for requests to external APIs
$weather_data = wp_cache_get('weather_data');
if (false === $weather_data) {
$weather_data = fetch_weather_data_from_api();
wp_cache_set('weather_data', $weather_data, 'api', 3600); // Cache for 1 hour
}
4. Image Optimization
Images can significantly increase page load times. PHP allows you to automate image optimization.
Code Example:
// Use the GD library to resize images
$image_path = 'path/to/image.jpg';
$thumbnail = image_resize($image_path, 300, 200, true);
// Convert images to WebP format for faster loading
if (function_exists('imagewebp')) {
$image_path = 'path/to/image.png';
$webp_image_path = 'path/to/image.webp';
$source_image = imagecreatefrompng($image_path);
imagewebp($source_image, $webp_image_path, 80);
}
Conclusion
Optimizing WordPress performance using PHP is an essential aspect of creating fast and responsive websites. In this article, we have covered some methods of caching, database optimization, query optimization, and image optimization with code examples for each. Use these tips to make your WordPress site more efficient and faster for users.
Creating websites using the WordPress platform and the PHP programming language is an engaging and powerful endeavor that allows you to build functional and creative web applications. In this article, we will explore the basics of website development using WordPress and PHP, along with providing code examples for better understanding.
Part 1: WordPress Basics
WordPress is one of the most popular platforms for creating websites and blogs. Its flexibility and user-friendliness make it an excellent choice for novice developers. Here are a few key concepts:
1.1 Installing WordPress:
The first step is to install WordPress on your hosting. Follow your hosting provider’s instructions or consult the WordPress documentation for installation guidance.
1.2 Themes and Plugins:
Themes define the appearance of your website, while plugins add additional functionality. You can choose from ready-made themes and plugins or create your own.
1.3 Creating Pages and Posts:
WordPress allows you to create static pages and blog posts. You can use the visual editor or HTML/PHP to create content.
Part 2: PHP Basics for WordPress
PHP is a server-side programming language used to create dynamic web pages. You’ll need some basic PHP knowledge to work with WordPress.
2.1 Built-in WordPress Functions:
WordPress provides a plethora of built-in functions to help you manage content and site functionality. For instance, get_header()
is used to display the site header, and the_title()
is used to display the title of a post.
2.2 Working with the Database:
WordPress stores data, such as posts and users, in a database. You can use wpdb
functions to execute database queries.
Example:
global $wpdb;
$results = $wpdb->get_results("SELECT * FROM {$wpdb->prefix}posts WHERE post_type = 'post'");
foreach ($results as $post) {
echo $post->post_title;
}
2.3 Creating Custom Shortcodes:
Shortcodes allow you to insert predefined content blocks into posts and pages. Here’s how you can create your own shortcode:
function custom_shortcode() {
return '<div class="custom-box">Hello, world!</div>';
}
add_shortcode('custom', 'custom_shortcode');
Part 3: Example WordPress Website Development using PHP
Let’s consider an example of creating a simple WordPress theme using PHP:
3.1 Theme Creation:
Create a new directory in wp-content/themes/
and name it my-custom-theme
. Inside, create a style.css
file with theme information.
Example style.css
:
/*
Theme Name: My Custom Theme
Description: Example custom theme for WordPress
Version: 1.0
Author: Your Name
*/
3.2 Creating the Main Theme File:
Create an index.php
file in your theme directory and add the following code:
<?php get_header(); ?>
<div id="content">
<?php if (have_posts()) : while (have_posts()) : the_post(); ?>
<div class="post">
<h2><?php the_title(); ?></h2>
<div class="post-content">
<?php the_content(); ?>
</div>
</div>
<?php endwhile; endif; ?>
</div>
<?php get_footer(); ?>
3.3 Styling and Images:
Create a css
directory within your theme and add a styles.css
file. You can also add images to the images
directory.
3.4 Activating the Theme:
In the WordPress admin panel, navigate to «Appearance» -> «Themes» and activate your new theme.
Conclusion:
This is just the beginning of an introduction to website development with WordPress and PHP. However, you’ve already mastered the basics of creating a WordPress theme and using PHP to manage content and functionality. Moving forward, you can explore more advanced concepts such as creating custom plugins, working with AJAX, and much more. Best of luck on your journey into the world of web development!
WordPress is one of the most popular platforms for building websites, and its flexibility allows developers to create various types of content. In this article, we will walk you through creating custom post types on your WordPress site using the PHP programming language. Custom post types allow you to organize content in a way that best suits your needs.
Introduction to Custom Post Types
The default post types in WordPress, such as «Posts» and «Pages,» work well for most websites. However, sometimes you may need a more specific type of content. For example, custom post types are perfect for portfolios, products, events, and more.
Advantages of using custom post types:
- Content Organization: Custom post types allow you to logically organize diverse content on your website.
- Ease of Management: Each custom post type has its own administrative panel, making it easy to add, edit, and delete content.
- Flexibility: You can define custom fields and taxonomies for your custom post types, tailoring them to your project’s specific requirements.
Creating Custom Post Types
Let’s start by creating a simple custom post type for a portfolio. Suppose you have a web agency and want to showcase your projects. You’ll need a post type called «Projects.»
Step 1: Adding Code to functions.php
Open your theme’s functions.php
file and add the following code:
// Register a custom post type for managing projects
function create_custom_post_type() {
$labels = array(
'name' => _x( 'Projects', 'post type general name', 'your-text-domain' ), // General name for the post type (plural)
'singular_name' => _x( 'Project', 'post type singular name', 'your-text-domain' ), // Singular name for the post type
'menu_name' => _x( 'Projects', 'admin menu', 'your-text-domain' ), // Menu name in the admin panel
'name_admin_bar' => _x( 'Project', 'add new on admin bar', 'your-text-domain' ), // Name displayed on admin bar
'add_new' => _x( 'Add New', 'project', 'your-text-domain' ), // Text for "Add New" button
'add_new_item' => __( 'Add New Project', 'your-text-domain' ), // Text for adding a new project
'new_item' => __( 'New Project', 'your-text-domain' ), // Text for a new project
'edit_item' => __( 'Edit Project', 'your-text-domain' ), // Text for editing a project
'view_item' => __( 'View Project', 'your-text-domain' ), // Text for viewing a project
'all_items' => __( 'All Projects', 'your-text-domain' ), // Text for "All Items" menu
'search_items' => __( 'Search Projects', 'your-text-domain' ), // Text for searching projects
'parent_item_colon' => __( 'Parent Projects:', 'your-text-domain' ), // Text for parent project
'not_found' => __( 'No projects found.', 'your-text-domain' ), // Text if no projects are found
'not_found_in_trash' => __( 'No projects found in Trash.', 'your-text-domain' ) // Text if no projects are found in the trash
);
$args = array(
'labels' => $labels, // Array of labels for the post type
'public' => true, // Whether the post type is publicly queryable and accessible
'publicly_queryable' => true, // Enable queries for this post type
'show_ui' => true, // Show UI in admin panel
'show_in_menu' => true, // Display in admin menu
'query_var' => true, // Enable query variables for this post type
'rewrite' => array( 'slug' => 'projects' ), // Permalink settings
'capability_type' => 'post', // Type of user permissions to handle posts
'has_archive' => true, // Enable archive page
'hierarchical' => false, // Posts will behave like flat items, not hierarchical
'menu_position' => null, // Position in admin menu
'menu_icon' => 'dashicons-portfolio', // Icon for the admin menu (see https://developer.wordpress.org/resource/dashicons/)
'supports' => array( 'title', 'editor', 'thumbnail', 'excerpt', 'custom-fields' ), // Supported features
'taxonomies' => array( 'category', 'post_tag' ), // Taxonomies associated with the post type
'show_in_rest' => true, // Enable REST API and Gutenberg editor support
);
// Register the new post type
register_post_type( 'projects', $args );
}
add_action( 'init', 'create_custom_post_type' );
This code uses the register_post_type
function, which allows you to define a new custom post type. In this case, we’re creating a post type called «Projects» with specific parameters.
Step 2: Updating Permalinks
To activate the new post type, you’ll need to update the permalinks. Simply go to «Settings» -> «Permalinks» and click «Save Changes.»
Step 3: Viewing the Results
After completing the above steps, you can navigate to the WordPress admin panel and see the new «Projects» section. Now you can create, edit, and delete your projects just like you would with regular posts and pages.
Step 4: Retrieving Data from Custom Post Types
To display data from the new custom post type on your website, you can use the following code:
$args = array(
'post_type' => 'projects',
'posts_per_page' => -1, // Show all projects
);
$projects_query = new WP_Query($args);
if ($projects_query->have_posts()) {
while ($projects_query->have_posts()) {
$projects_query->the_post();
// Display project data
echo '<h2>' . get_the_title() . '</h2>';
echo '<div>' . get_the_content() . '</div>';
// Additional fields, if any
}
wp_reset_postdata();
} else {
echo 'No projects found.';
}
In this example, we use WP_Query
to retrieve all posts of the «Projects» type. We then loop through each post and display the title and content of the project. You can also add the output of additional fields if you have defined them.
Conclusion
Creating custom post types is a powerful tool that allows you to tailor your website’s structure to your needs. In this article, we covered the basic steps of creating a custom post type «Projects» for a portfolio, as well as how to retrieve and display data from this post type on your website.
Use this knowledge to create unique and flexible content on your WordPress site!