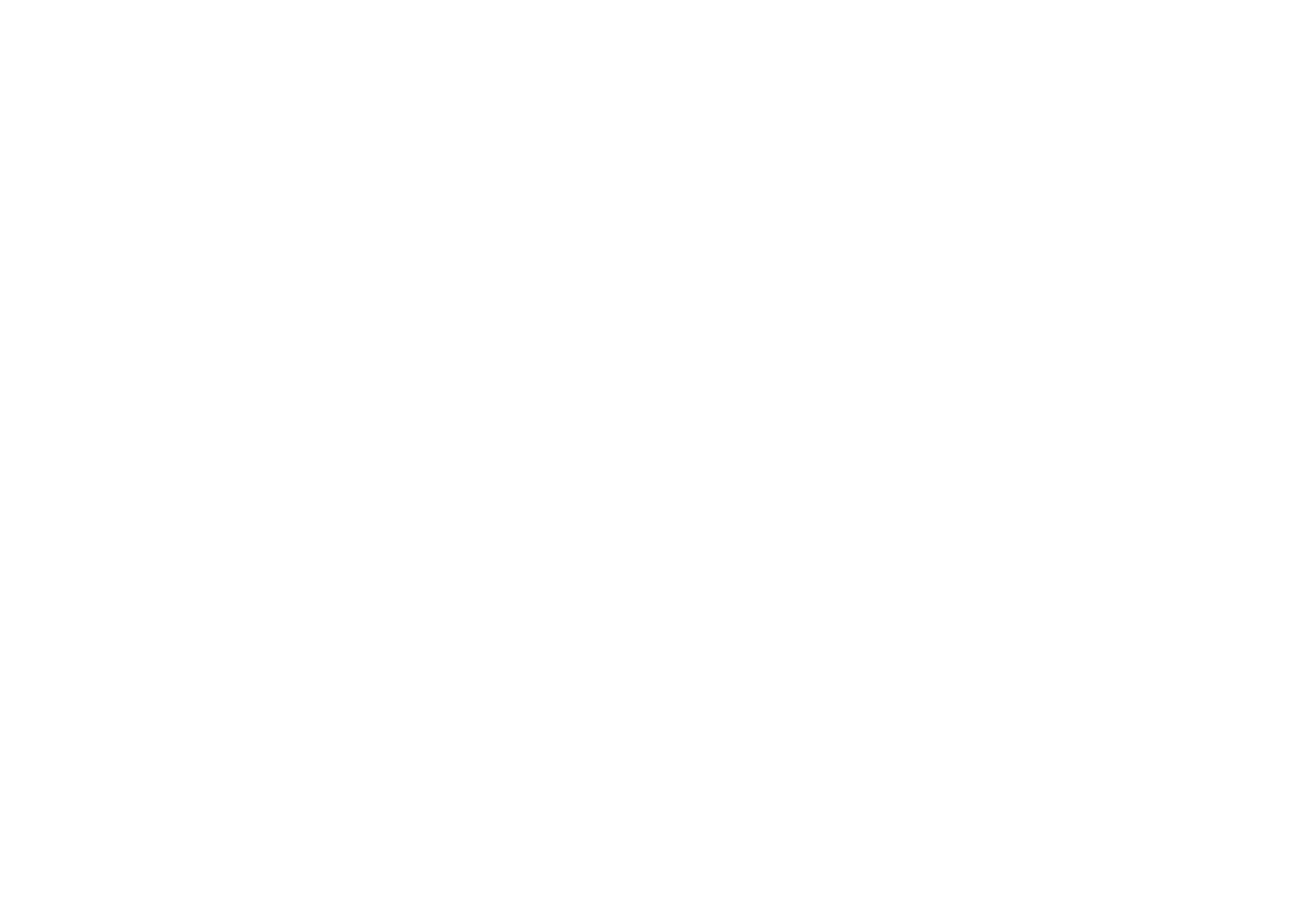
Integrating Databases into Web Applications using PHP and WordPress
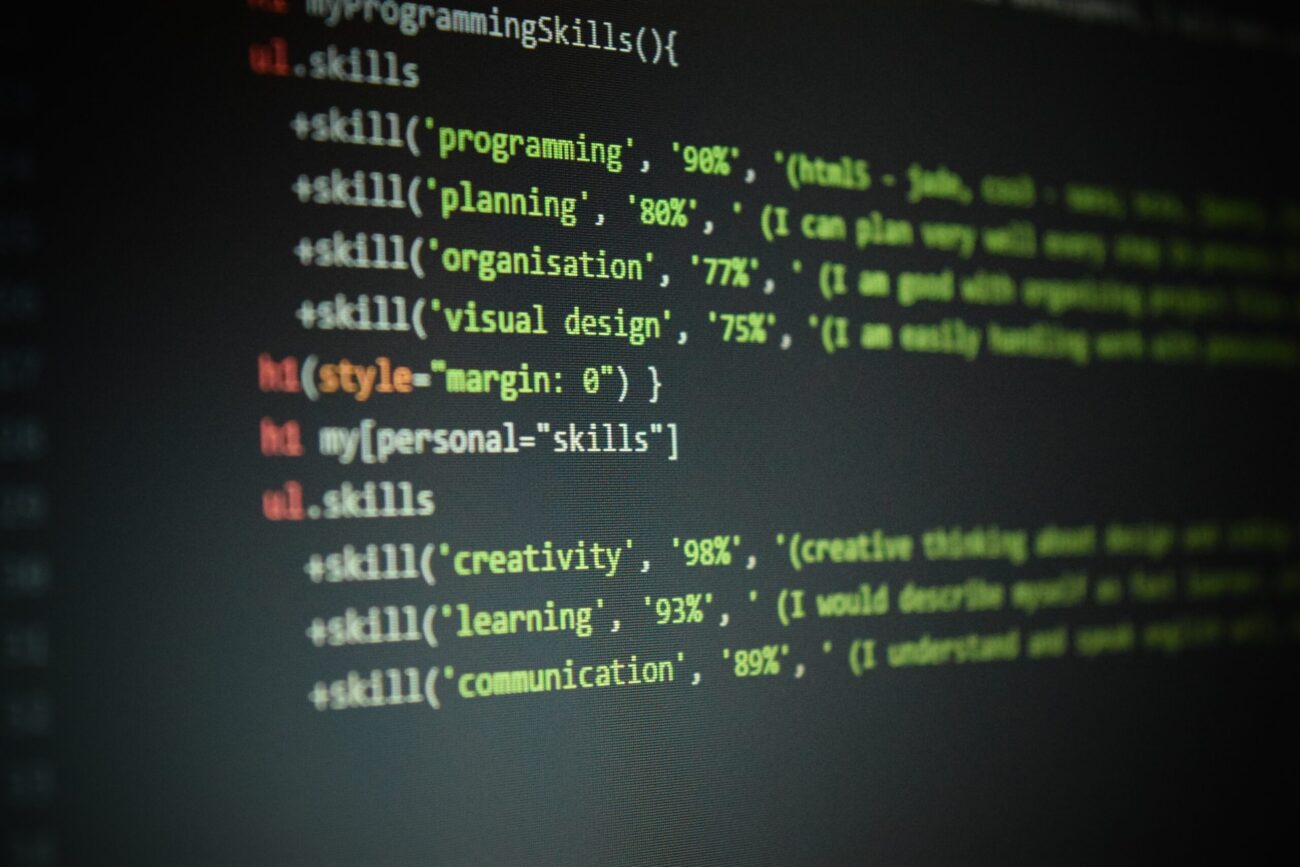
In the modern world of web development, interacting with databases has become an integral part of creating powerful and functional web applications. One of the most popular tools for developing dynamic websites and applications is PHP. When combined with the widely used content management platform WordPress, PHP offers developers a multitude of opportunities for integrating databases. In this article, we will explore methods of integrating databases into web applications using PHP and WordPress, provide code examples, and explain each step of the process in detail.
Part 1: Basics of Working with Databases
Before diving into integrating databases into PHP and WordPress, let’s understand the basics of working with databases. A database is a structured repository of information that can be efficiently managed and retrieved. To interact with a database, we will use the Structured Query Language (SQL).
Part 2: Creating and Configuring the Database
Before we can begin integrating the database into our web application, we need to create and configure the database. For our example, let’s consider creating a simple database to store user information for our web application. We will use MySQL, one of the most popular database management systems.
Code Example SQL:
CREATE DATABASE myapp_db;
USE myapp_db;
CREATE TABLE users (
id INT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) NOT NULL,
password VARCHAR(255) NOT NULL
);
Part 3: Connecting to the Database
Now that the database is created, let’s learn how to establish a connection to it from PHP code. We will use the PHP Data Objects (PDO) extension, which provides an abstraction layer for accessing different databases.
Code Example PHP:
$dsn = "mysql:host=localhost;dbname=myapp_db";
$username = "username";
$password = "password";
try {
$pdo = new PDO($dsn, $username, $password);
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "Connected to the database";
} catch (PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
Part 4: Executing Database Queries
After successfully connecting to the database, we can execute queries to add, update, and retrieve data. Let’s look at examples of executing different queries using PDO.
Code Example PHP:
// Inserting a new user
$query = "INSERT INTO users (username, email, password) VALUES (?, ?, ?)";
$stmt = $pdo->prepare($query);
$username = "newuser";
$email = "newuser@example.com";
$password = password_hash("password123", PASSWORD_DEFAULT);
$stmt->execute([$username, $email, $password]);
echo "New user added";
// Retrieving a list of users
$query = "SELECT * FROM users";
$stmt = $pdo->query($query);
while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) {
echo "ID: " . $row['id'] . ", Name: " . $row['username'] . ", Email: " . $row['email'] . "<br>";
}
Part 5: Integration with WordPress
Next, we’ll move on to integrating the database with WordPress, a popular content management platform. WordPress provides its own methods for working with databases, making interaction easier and ensuring data security.
Code Example:
global $wpdb;
// Inserting a new record into a WordPress table
$table_name = $wpdb->prefix . 'custom_table';
$data = array('name' => 'John', 'email' => 'john@example.com');
$wpdb->insert($table_name, $data);
echo "New record added";
// Retrieving data from a WordPress table
$results = $wpdb->get_results("SELECT * FROM $table_name");
foreach ($results as $result) {
echo "ID: " . $result->id . ", Name: " . $result->name . ", Email: " . $result->email . "<br>";
}
Conclusion
Integrating databases into web applications using PHP and WordPress is a key aspect of developing modern dynamic websites and applications. In this article, we have covered the basics of working with databases, creating and configuring a database, connecting to it from PHP code, executing queries, and integrating with WordPress. Mastering these concepts will enable you to develop powerful and efficient web applications capable of working with data and providing an enhanced user experience.