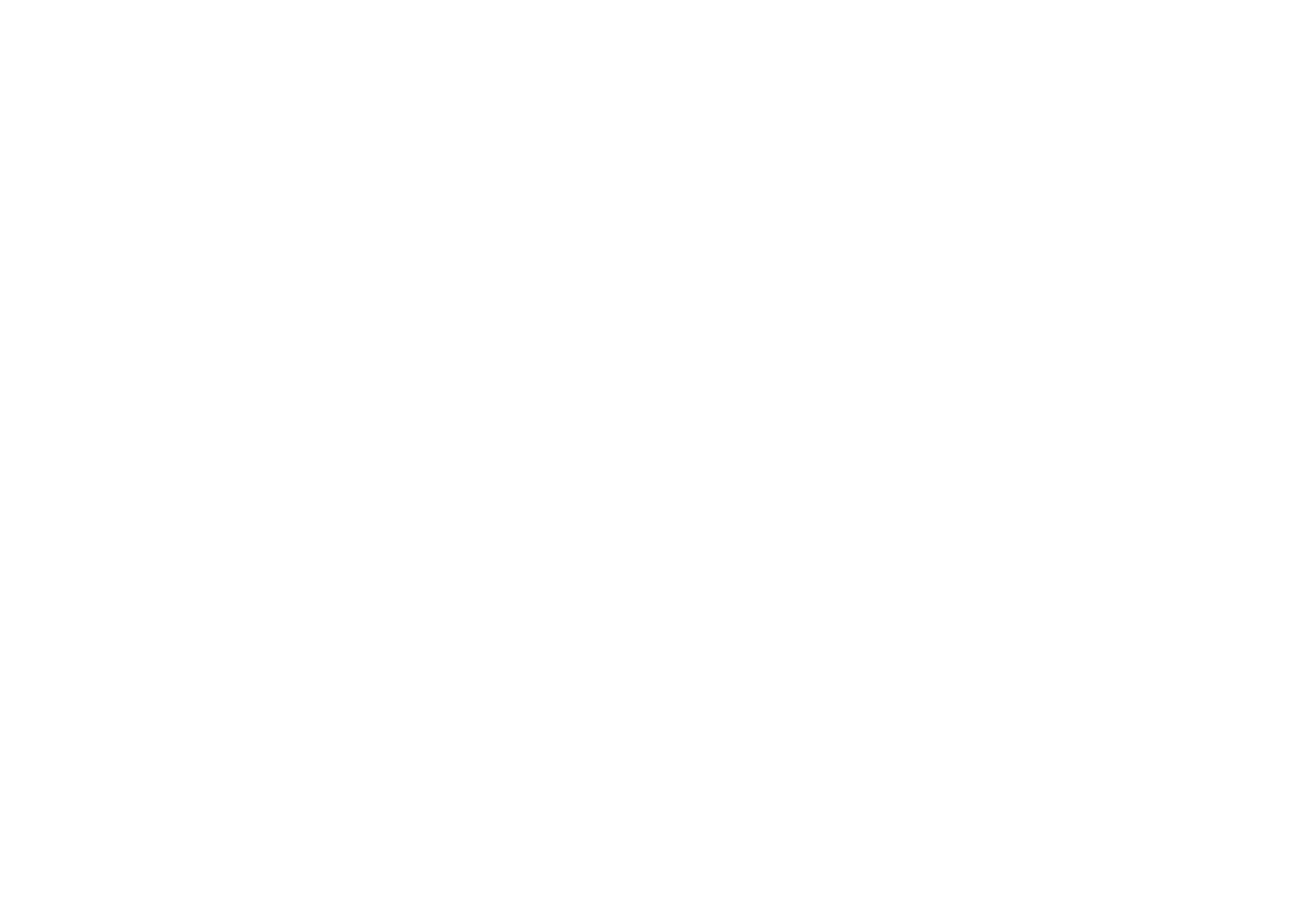
WordPress Plugin Development Using PHP: Comprehensive Guide
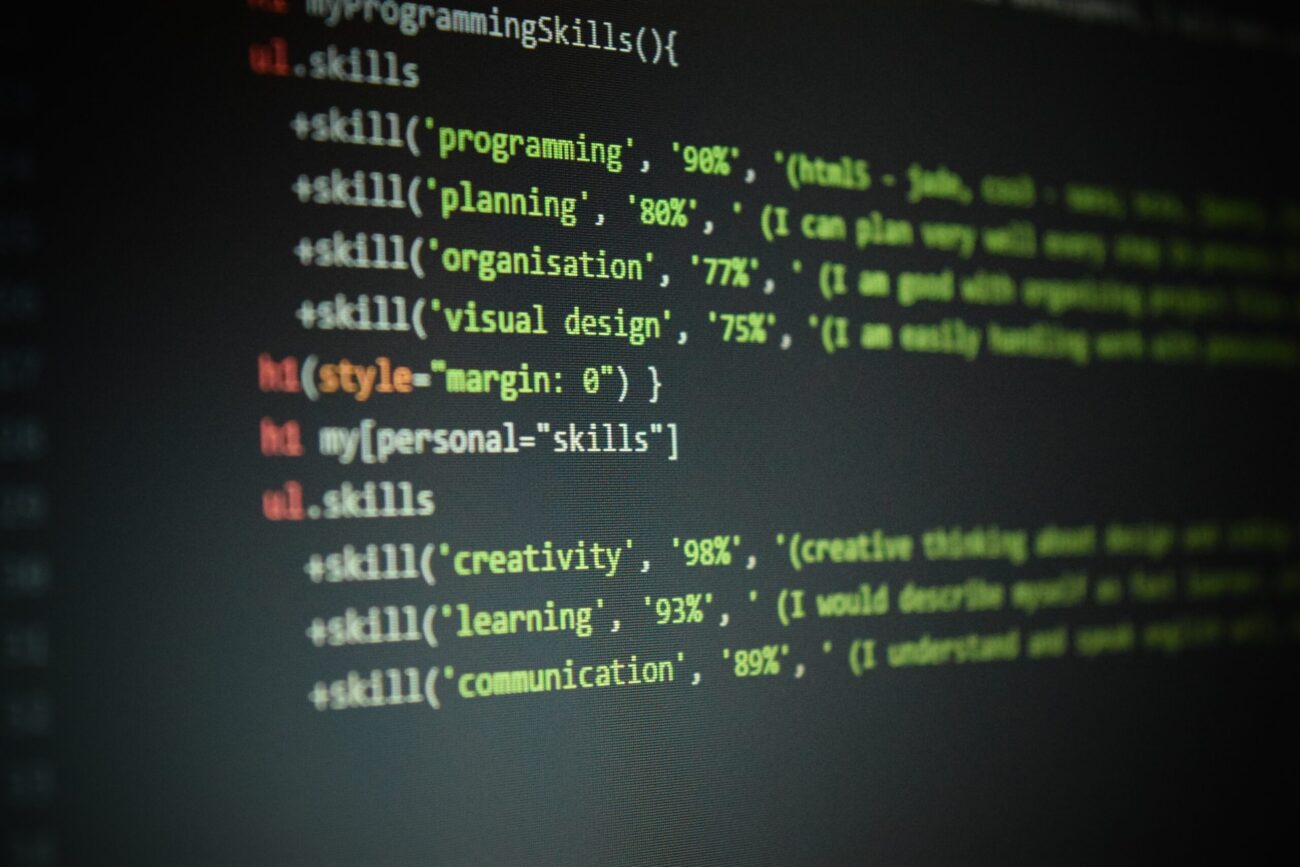
Creating plugins for WordPress using the PHP programming language is an essential part of extending the functionality of the WordPress platform. In this article, we will discuss key aspects of plugin development and provide you with a solid understanding of how to embark on this exciting process.
Part 1: Fundamentals of WordPress Plugin Development
1. Preparation for Development:
Before diving into plugin creation, it’s important to set up your development environment. Install WordPress on a local server or use an online development environment. Create a separate folder within the wp-content/plugins
directory for your plugin.
2. Creating a Simple Plugin:
Let’s start by creating a minimal plugin. In your plugin’s folder, create a file named my-plugin.php
and add basic information:
<?php
/*
Plugin Name: My Plugin
Description: Example simple plugin for WordPress.
Version: 1.0
Author: Your Name
*/
This establishes a starting point for your plugin.
Part 2: Extending Functionality
3. Adding a New Admin Menu Item:
Let’s add a new item to the WordPress admin menu. Use the following code:
function my_plugin_menu() {
add_menu_page(
'My Page',
'My Page',
'manage_options',
'my-plugin-slug',
'my_plugin_page'
);
}
function my_plugin_page() {
echo '<div class="wrap"><h2>My Page</h2><p>Hello, world!</p></div>';
}
add_action('admin_menu', 'my_plugin_menu');
Part 3: Interacting with the Database
4. Creating a Database Table:
Plugins often require data storage. Let’s create a table in the database:
function create_custom_table() {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_table';
$charset_collate = $wpdb->get_charset_collate();
$sql = "CREATE TABLE $table_name (
id INT NOT NULL AUTO_INCREMENT,
name VARCHAR(100) NOT NULL,
email VARCHAR(100) NOT NULL,
PRIMARY KEY (id)
) $charset_collate;";
require_once(ABSPATH . 'wp-admin/includes/upgrade.php');
dbDelta($sql);
}
register_activation_hook(__FILE__, 'create_custom_table');
5. Interacting with the Database:
Let’s continue by adding functions for data insertion and retrieval:
function insert_data($name, $email) {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_table';
$wpdb->insert(
$table_name,
array(
'name' => $name,
'email' => $email,
)
);
}
function get_data() {
global $wpdb;
$table_name = $wpdb->prefix . 'custom_table';
$results = $wpdb->get_results("SELECT * FROM $table_name");
return $results;
}
Part 4: Creating a Custom Widget
6. Creating a Custom Widget:
Plugins can also include custom widgets. Let’s create a widget that displays a list of «Products»:
class Product_Widget extends WP_Widget {
function __construct() {
parent::__construct(
'product_widget',
'Product Widget',
array('description' => 'Displays a list of products')
);
}
public function widget($args, $instance) {
echo $args['before_widget'];
echo $args['before_title'] . 'Products' . $args['after_title'];
$products = get_data();
echo '<ul>';
foreach ($products as $product) {
echo '<li>' . $product->name . '</li>';
}
echo '</ul>';
echo $args['after_widget'];
}
}
function register_product_widget() {
register_widget('Product_Widget');
}
add_action('widgets_init', 'register_product_widget');
Conclusion:
This article provided you with an introduction to WordPress plugin development using PHP. We covered the basics of creating a plugin, adding a new admin menu page, interacting with the database, and creating a custom widget. Plugin development is a powerful way to customize and extend your WordPress website’s functionality. Use this knowledge to create innovative solutions and enhance your projects.