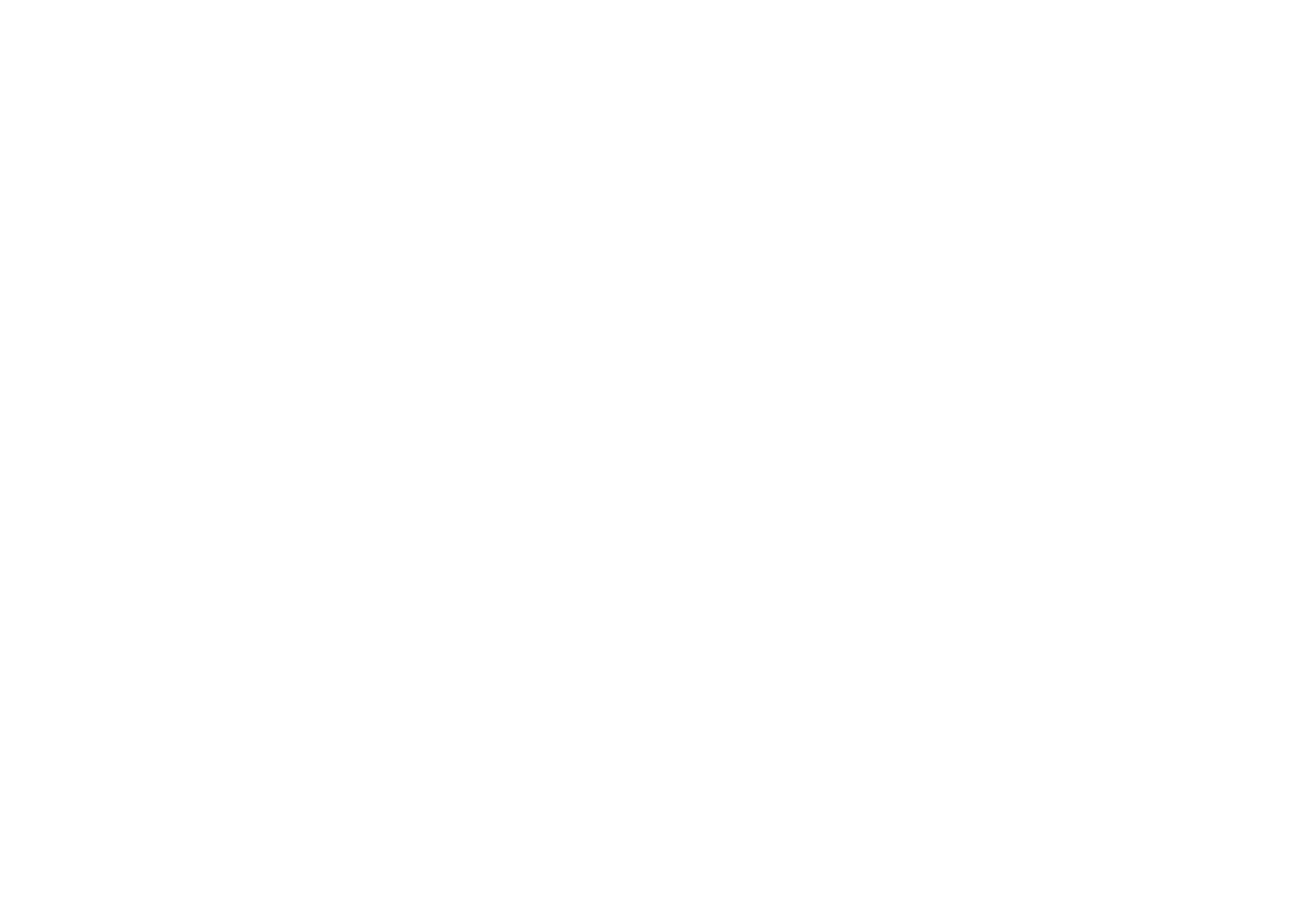
Working with APIs in WordPress and PHP: Expanding Your Website's Capabilities
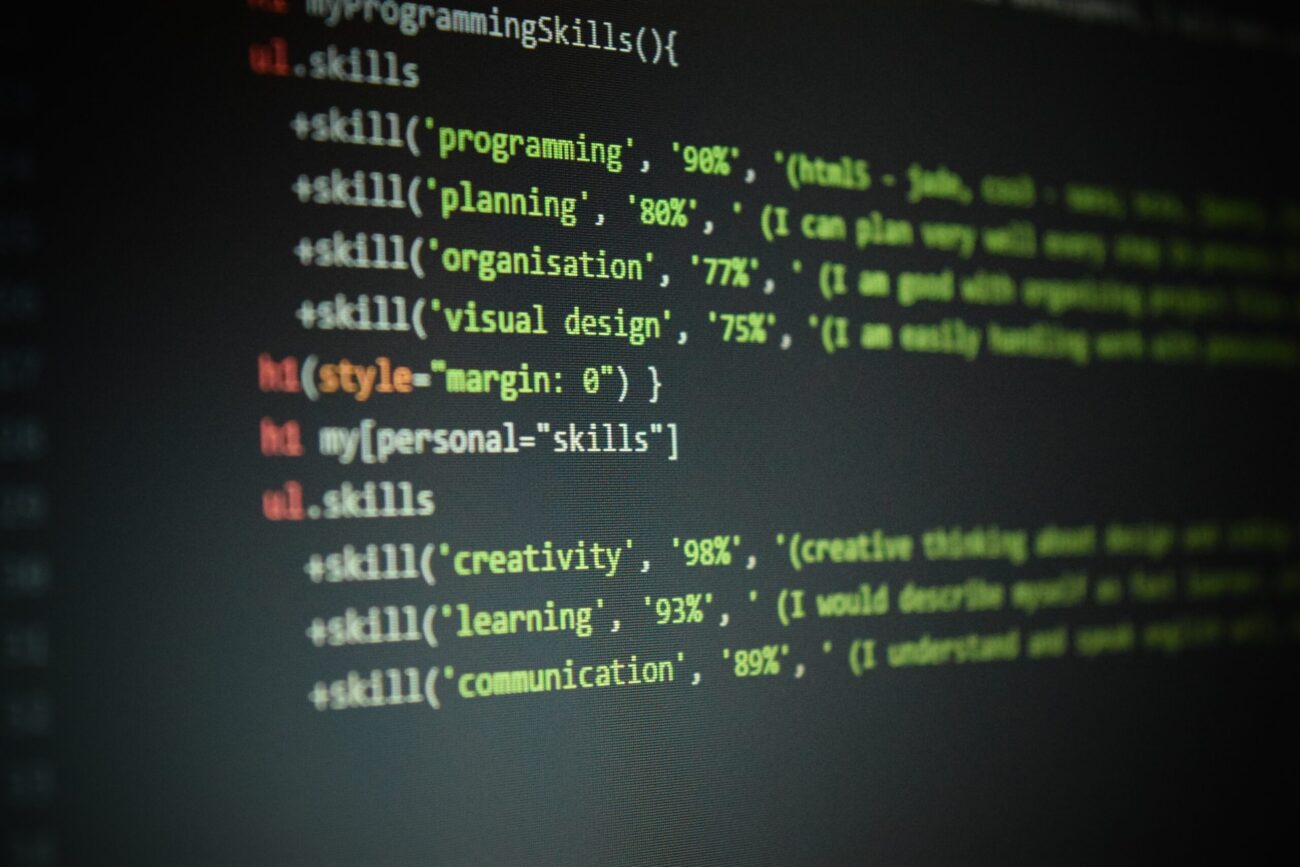
An API (Application Programming Interface) is a set of instructions and protocols that allow different programs to interact with each other. In the case of WordPress, an API enables you to create, modify, and retrieve data from your website using other applications or services. In this article, we will explore how to work with APIs in WordPress and PHP to extend the functionality of your website.
Introduction to the WordPress API
WordPress provides several different APIs for interacting with your website. One of the key APIs is the REST API, which allows you to send and receive data from your site using standard HTTP methods such as GET, POST, PUT, and DELETE. The REST API opens up vast possibilities for integrating your site with other applications and services.
Authentication and Obtaining an API Key
Before you start using the REST API, you’ll need an API key. To do this, go to the WordPress admin panel and navigate to «Settings» -> «General». Scroll down and find the «REST API» section. Here, you can create and manage API keys.
After creating the key, remember to keep it secure as it will be used for authentication in your API requests.
Examples of Using the REST API
Let’s consider several examples of using the REST API in WordPress.
Getting a List of Posts
To retrieve a list of all posts from your site, you can make a GET request as follows:
$api_url = 'https://your_site/wp-json/wp/v2/posts';
$response = wp_remote_get( $api_url );
$data = wp_remote_retrieve_body( $response );
$posts = json_decode( $data );
Creating a New Post
To create a new post on your site, you can use a POST request:
$api_url = 'https://your_site/wp-json/wp/v2/posts';
$data = array(
'title' => 'New Post',
'content' => 'Content of the new post...',
'status' => 'publish'
);
$response = wp_remote_post( $api_url, array(
'headers' => array(
'Authorization' => 'Bearer your_api_key',
),
'body' => json_encode( $data )
));
Updating a Post
To update an existing post, you can use a PUT request:
$post_id = 123; // ID of the post to update
$api_url = 'https://your_site/wp-json/wp/v2/posts/' . $post_id;
$data = array(
'title' => 'New Post Title',
'content' => 'New content of the post...'
);
$response = wp_remote_request( $api_url, array(
'method' => 'PUT',
'headers' => array(
'Authorization' => 'Bearer your_api_key',
),
'body' => json_encode( $data )
));
Deleting a Post
To delete a post, use a DELETE request:
$post_id = 123; // ID of the post to delete
$api_url = 'https://your_site/wp-json/wp/v2/posts/' . $post_id;
$response = wp_remote_request( $api_url, array(
'method' => 'DELETE',
'headers' => array(
'Authorization' => 'Bearer your_api_key',
)
));
Using PHP for API Interaction
In addition to the WordPress REST API, PHP offers a multitude of built-in functions for working with external APIs. Let’s explore a few examples.
Sending a GET Request
To send a GET request to an external API, you can use the file_get_contents
function:
$api_url = 'https://api.example.com/data';
$response = file_get_contents( $api_url );
$data = json_decode( $response );
Sending a POST Request
For sending a POST request, you can utilize the curl
function:
$api_url = 'https://api.example.com/create';
$data = array(
'param1' => 'value1',
'param2' => 'value2'
);
$ch = curl_init( $api_url );
curl_setopt( $ch, CURLOPT_POST, 1 );
curl_setopt( $ch, CURLOPT_POSTFIELDS, http_build_query( $data ) );
curl_setopt( $ch, CURLOPT_RETURNTRANSFER, true );
$response = curl_exec( $ch );
curl_close( $ch );
Authenticating with an External API
If authentication is required for an external API, you can pass necessary headers:
$api_url = 'https://api.example.com/data';
$headers = array(
'Authorization: Bearer your_api_key',
'Content-Type: application/json'
);
$options = array(
'http' => array(
'header' => implode( "\r\n", $headers )
)
);
$context = stream_context_create( $options );
$response = file_get_contents( $api_url, false, $context );
$data = json_decode( $response );
Conclusion
Working with APIs in WordPress and PHP offers vast opportunities for extending your website’s functionality. You can retrieve data, create and update content, as well as integrate your site with other applications and services. Thanks to the convenience and versatility of APIs, your website can interact seamlessly with the digital ecosystem, providing a richer user experience and opening doors to innovative possibilities.